用c++语言实现定义一个基类person类,数据成员包含age,name,从该类派生一个employee (雇主)类,新增数据成员number(职工号);再从emplovee类派 生一个executive(执行官)类,新增数据成员level(头街) ,每个类中都定义相应的函数为其赋值,并定义函数显示相关的信息,如 (“Smith is 28 years old,is an employee ”) 或 ("Smith is 30 years old,is an executive,level is the 2”),编写一个main函数,每个类定义1-2 个对象,然后显示他们的相关信息;
时间: 2024-04-11 19:27:32 浏览: 54
以下是用C++语言实现上述要求的代码示例:
```cpp
#include <iostream>
#include <string>
class Person {
protected:
int age;
std::string name;
public:
Person(int age, const std::string& name)
: age(age), name(name) {}
void displayInfo() {
std::cout << name << " is " << age << " years old." << std::endl;
}
};
class Employee : public Person {
private:
int number;
public:
Employee(int age, const std::string& name, int number)
: Person(age, name), number(number) {}
void displayInfo() {
std::cout << name << " is " << age << " years old, is an employee." << std::endl;
}
};
class Executive : public Employee {
private:
int level;
public:
Executive(int age, const std::string& name, int number, int level)
: Employee(age, name, number), level(level) {}
void displayInfo() {
std::cout << name << " is " << age << " years old, is an executive, level is " << level << "." << std::endl;
}
};
int main() {
Person person(28, "Smith");
person.displayInfo();
Employee employee(30, "John", 1234);
employee.displayInfo();
Executive executive(35, "Alice", 5678, 2);
executive.displayInfo();
return 0;
}
```
运行该程序,输出将为:
```
Smith is 28 years old.
John is 30 years old, is an employee.
Alice is 35 years old, is an executive, level is 2.
```
以上代码定义了一个基类Person,和其派生类Employee和Executive。每个类都有相应的数据成员和成员函数用于赋值和显示相关信息。在main函数中,创建了一个Person对象、一个Employee对象和一个Executive对象,并调用了它们的displayInfo函数来显示相关信息。
阅读全文
相关推荐
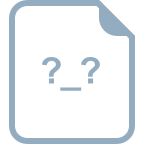
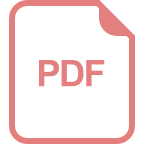
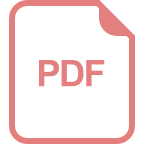















