利用循环数组q[m]储存队列,该队列只有一个队列头指针front,不设队列尾指针,而设一个计数器count,用以记录队列中元素的个数。 实现队列的判空、判满、入队和出队操作c++代码
时间: 2024-09-23 18:15:14 浏览: 14
在 C++ 中,利用循环数组 `q[m]` 存储队列并且只维护一个队列头指针 `front` 和一个计数器 `count` 可以简化队列的实现。由于不设置队尾指针,我们需要考虑队列索引的循环性质。以下是对应的 C++ 代码实现:
```cpp
#include <iostream>
const size_t MAX_SIZE = 100; // 设置队列最大容量
class CircularQueue {
private:
int q[MAX_SIZE]; // 循环数组
size_t front; // 队首指针
size_t rear; // 为了计算索引的实际位置,但并不实际使用
size_t count; // 当前元素个数
public:
CircularQueue() : front(0), rear(0), count(0) {}
// 判空
bool isEmpty() const {
return count == 0;
}
// 判满
bool isFull() const {
return count == MAX_SIZE;
}
// 入队操作
void enqueue(int value) {
if (isFull()) {
throw std::runtime_error("Queue is full");
}
q[rear] = value;
rear = (rear + 1) % MAX_SIZE; // 循环索引
count++;
}
// 出队操作
int dequeue() {
if (isEmpty()) {
throw std::runtime_error("Queue is empty");
}
int removedValue = q[front];
front = (front + 1) % MAX_SIZE; // 循环索引
count--;
return removedValue;
}
// 获取队首元素,不修改队列状态
int getFront() const {
if (isEmpty()) {
throw std::runtime_error("Queue is empty");
}
return q[front];
}
};
int main() {
CircularQueue cq;
try {
cq.enqueue(1);
cq.enqueue(2);
cq.enqueue(3);
std::cout << "Front element: " << cq.getFront() << std::endl;
std::cout << "Queue after enqueuing and getting front: " << std::endl;
cq.display();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
// 仅用于显示队列元素(非必要)
void CircularQueue::display() {
for (size_t i = front; i != rear; i = (i + 1) % MAX_SIZE) {
std::cout << q[i] << " ";
}
std::cout << "\n";
}
相关推荐
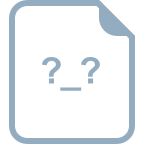
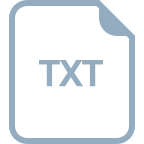
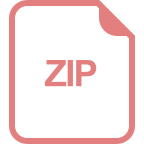













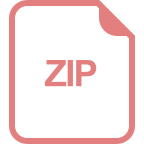