编写算法,统计顺序表中元素e的个数
时间: 2024-10-21 12:03:13 浏览: 23
编写一个简单的算法来统计顺序表(数组或链表)中元素e的个数,你可以按照以下步骤:
1. **初始化计数器**:创建一个整型变量count并将其值设为0,用于记录元素e出现的次数。
2. **遍历列表**:
- 如果当前元素等于e,将count加一。
- 使用循环结构,如for循环(数组)或迭代(链表),直到遍历完整个列表。
3. **返回结果**:
- 遍历结束后,count就是元素e在列表中出现的次数,返回这个值。
**伪代码示例(数组)**:
```plaintext
function count_element_in_array(array, e):
count = 0
for element in array:
if element == e:
count += 1
return count
```
**伪代码示例(链表)**:
```plaintext
function count_element_in_linked_list(head, e):
current = head
while current is not None:
if current.data == e:
count += 1
current = current.next
return count
```
相关问题
编写算法,统计顺序表中元素e的个数,使用c语言
在C语言中,编写一个简单的算法来统计顺序表(数组)中元素`e`的个数,你可以创建一个函数,遍历整个列表并计数。这里是一个基本示例:
```c
#include <stdio.h>
// 定义顺序表结构体
typedef struct {
int data; // 数据域
} Node;
// 功能:统计顺序表中元素e的个数
int countOccurrences(Node* list, int n, int e) {
int count = 0;
for (int i = 0; i < n; i++) { // 遍历数组
if (list[i].data == e) {
count++; // 如果找到e,计数加一
}
}
return count;
}
int main() {
int elements[] = {1, 2, 3, 4, 5, 2, 6, 2}; // 示例顺序表
int size = sizeof(elements) / sizeof(elements[0]);
int target = 2; // 要查找的目标元素
int occurrences = countOccurrences(elements, size, target);
printf("元素 %d 出现了 %d 次.\n", target, occurrences);
return 0;
}
```
在这个程序中,`countOccurrences`函数接收一个指向顺序表首节点的指针、列表长度以及目标元素值。它会返回该元素在列表中出现的次数。
编写算法,实现顺序表中元素的逆置,并在应用程序中调用显示结果;在顺序表中查找值为 a 的元素的位置(可能不只一处)。
要实现顺序表中元素的逆置,你可以编写一个函数来交换顺序表的首尾元素,直到达到中间位置。以下是一个实现逆置的C语言函数示例:
```c
#include <stdio.h>
// 函数原型声明
void reverse(int arr[], int n);
int find(int arr[], int n, int a);
int main() {
int array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9}; // 示例顺序表
int n = sizeof(array) / sizeof(array[0]); // 数组元素的个数
int a = 5; // 要查找的值
// 逆置顺序表
reverse(array, n);
// 显示逆置后的顺序表
for (int i = 0; i < n; ++i) {
printf("%d ", array[i]);
}
printf("\n");
// 查找值为a的元素的位置
int positions[10]; // 假设不会超过10个相同的元素
int count = 0;
int position = find(array, n, a);
// 打印所有找到的位置
while (position != -1) {
positions[count++] = position;
position = find(array, n, a);
}
// 打印位置信息
printf("Element %d found at positions: ", a);
for (int i = 0; i < count; ++i) {
printf("%d ", positions[i]);
}
printf("\n");
return 0;
}
// 逆置函数
void reverse(int arr[], int n) {
for (int i = 0; i < n / 2; ++i) {
int temp = arr[i];
arr[i] = arr[n - i - 1];
arr[n - i - 1] = temp;
}
}
// 查找函数
int find(int arr[], int n, int a) {
for (int i = 0; i < n; ++i) {
if (arr[i] == a) {
return i;
}
}
return -1; // 如果没有找到,返回-1
}
```
这段代码首先定义了两个函数:`reverse`用于逆置顺序表,`find`用于查找顺序表中某个值的位置。`main`函数中首先逆置了一个顺序表,然后打印逆置后的顺序表。接着调用`find`函数查找特定值`a`的所有出现位置,并打印出来。
阅读全文
相关推荐
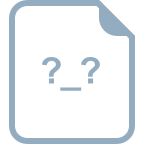
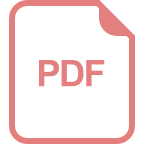
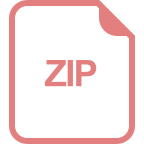

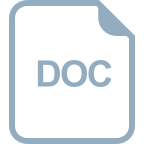
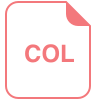
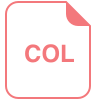










