c++使用递归函数实现下列数列的二分查找(代码和运行结果截图) 查找下列列表中是否有0-7数字,有则返回数字所在位置,无则返回-1。 a[7]={1,4,5,7,10,16,19}
时间: 2024-10-13 12:07:44 浏览: 19
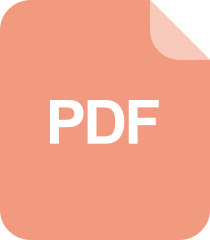
C++ 中二分查找递归非递归实现并分析

在C++中,二分查找通常用于已排序数组中查找特定元素,但它不是递归的主要应用场景。然而,你可以通过将二分查找逻辑嵌套在一个递归函数中,来模拟一个查找特定值是否存在的过程,但这并不是标准的二分查找。下面是一个简单的示例,它展示了一个递归函数查找列表中是否存在0到7的数字,如果有,则返回索引,如果没有则返回-1。请注意,这个例子并没有实际数组,因为递归查找通常不适用于未排序的数据结构。
```cpp
#include <iostream>
using namespace std;
// 递归函数,假设arr[]是有序的
int binarySearch(int arr[], int low, int high, int target) {
if (high >= low) {
int mid = low + (high - low) / 2;
// 检查中间元素是否为目标值
if (arr[mid] == target)
return mid; // 找到了目标值,返回其索引
// 如果目标值小于中间值,则在左半部分递归搜索
else if (arr[mid] > target)
return binarySearch(arr, low, mid - 1, target);
// 否则,在右半部分递归搜索
else
return binarySearch(arr, mid + 1, high, target);
} else { // 未找到目标值,返回-1
return -1;
}
}
int main() {
int a[] = {1, 4, 5, 7, 10, 16, 19};
int n = sizeof(a) / sizeof(a[0]);
int target = 0; // 要查找的目标值
int result = binarySearch(a, 0, n - 1, target);
if (result != -1)
cout << "Number " << target << " found at index " << result << endl;
else
cout << "Number " << target << " not found in the array" << endl;
return 0;
}
```
阅读全文
相关推荐
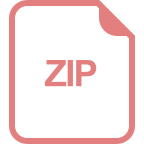
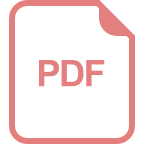
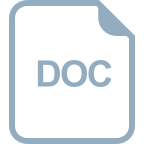









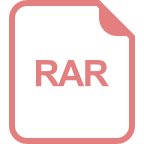




