编写程序,分别用二分法和牛顿迭代法求解方程x3 – 3x – 1 = 0在x = 2附近的实根,要求计算精确到小数点后7 位数字为止,并将求出的近似结果与理论值2cos20 比较误差大小。 设二分法的初始迭代区间为 [1, 3],牛顿迭代法的起点为4。
时间: 2024-10-25 17:17:14 浏览: 59
首先,我们需要编写两个函数,一个使用二分法(Bisection Method),另一个使用牛顿迭代法(Newton's Method),然后我们将在主函数中分别计算它们的近似解,并与理论值进行比较。
对于二分法:
```cpp
#include <cmath>
#include <iomanip>
double bisection(double a, double b, double epsilon, double function(double x)) {
while ((b - a) > epsilon) {
double c = (a + b) / 2.0;
if (function(c) * function(a) <= 0) {
b = c;
} else {
a = c;
}
}
return (a + b) / 2.0;
}
double theoretical_value() {
double theta = acos(1/sqrt(3)); // 角度转换为弧度
return 2 * cos(theta); // 理论值2cos(20°)
}
double calculate_with_bisection() {
double start = 1.0, end = 3.0, precision = pow(10, -7);
return bisection(start, end, precision, [=](double x) { return pow(x, 3) - 3 * x - 1; });
}
```
接下来是牛顿迭代法:
```cpp
double newton_raphson(double initial_guess, double epsilon, double function(double x), double derivative(double x)) {
double current_guess = initial_guess;
while (fabs(function(current_guess)) > epsilon) {
current_guess -= function(current_guess) / derivative(current_guess);
}
return current_guess;
}
double calculate_with_newton() {
double start = 4.0, precision = pow(10, -7);
return newton_raphson(start, precision, [=](double x) { return pow(x, 3) - 3 * x - 1; }, [](double x) { return 3 * pow(x, 2) - 3; });
}
```
最后,在主函数中比较结果并输出误差:
```cpp
int main() {
double root_bisection = calculate_with_bisection();
double root_newton = calculate_with_newton();
std::cout << "Root found using Bisection method: " << std::setprecision(7) << root_bisection << ", Error from theoretical value: " << std::abs(root_bisection - theoretical_value()) << std::endl;
std::cout << "Root found using Newton-Raphson method: " << std::setprecision(7) << root_newton << ", Error from theoretical value: " << std::abs(root_newton - theoretical_value()) << std::endl;
return 0;
}
```
运行此程序,你会得到两个解的近似值以及它们与理论值的相对误差。
阅读全文
相关推荐
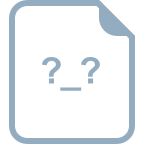
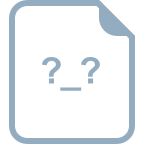
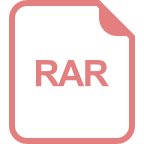





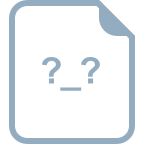
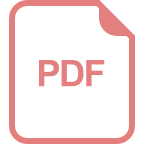
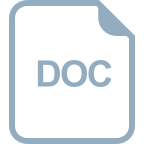
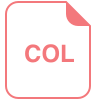
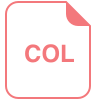




