c++快速排序生成998组随机数组
时间: 2024-12-17 16:24:27 浏览: 3
在 C++ 中,快速排序是一种常用的高效排序算法,用于生成一组随机数组可以结合 `<random>` 标准库来完成。以下是使用 C++11 或更高版本实现快速排序并生成998组随机整数数组的一个简短示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
// 快速排序函数
void quickSort(std::vector<int>& arr, int low, int high) {
if (low < high) {
// 找到基准元素
int pivotIndex = partition(arr, low, high);
// 对左右两个部分递归排序
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
// 分区函数
int partition(std::vector<int>& arr, int low, int high) {
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(low, high); // 随机选择基准
int pivotValue = dis(gen);
int pivotIndex = low;
for (int i = low; i < high; ++i) {
if (arr[i] < pivotValue) {
std::swap(arr[pivotIndex], arr[i]);
++pivotIndex;
}
}
std::swap(arr[pivotIndex], arr[high]);
return pivotIndex;
}
int main() {
const size_t numArrays = 998;
const size_t arraySize = 100; // 你可以根据需要调整数组大小
std::vector<std::vector<int>> randomArrays(numArrays, std::vector<int>(arraySize));
for (size_t i = 0; i < numArrays; ++i) {
std::generate(randomArrays[i].begin(), randomArrays[i].end(),
[](){ return std::rand(); }); // 使用 C++ 标准库生成随机数
// 对生成的随机数组进行排序
quickSort(randomArrays[i], 0, randomArrays[i].size() - 1);
}
// 现在 randomArrays 数组包含了998组随机排序后的整数数组
// 可以按需打印或处理这些数组
for (const auto& arr : randomArrays) {
for (int value : arr) {
std::cout << value << " ";
}
std::cout << "\n";
}
return 0;
}
```
在这个例子中,我们首先生成了998组大小为100的随机整数数组,然后对每个数组进行了快速排序。注意这里使用的是C++11的随机数生成,如果你使用的是旧版编译器,可能需要使用 `<cstdlib>` 库的 `std::srand` 和 `std::rand`。
阅读全文
相关推荐
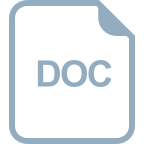
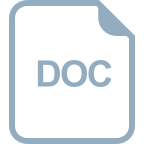
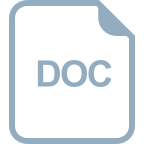
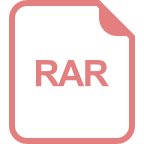














