对于给定的n个元素的数组a[0,n-1],要求从中找到第k小的元素java
时间: 2024-10-17 17:03:52 浏览: 26
在Java中,寻找一个整数数组a中的第k小元素(也称为“快速选择”或“三分查找法”的变种),可以使用分治策略实现。这里推荐一种名为“随机化快速选择算法”,它通常比直接的排序解决方案更有效率:
```java
import java.util.Random;
public class KthSmallestElement {
public static int findKthLargest(int[] nums, int k) {
if (nums == null || nums.length == 0 || k < 1 || k > nums.length) {
throw new IllegalArgumentException("Invalid input");
}
return quickSelect(nums, 0, nums.length - 1, k);
}
private static int partition(int[] nums, int low, int high) {
Random rand = new Random();
int pivotIndex = rand.nextInt(high - low + 1) + low;
int pivotValue = nums[pivotIndex];
swap(nums, pivotIndex, high); // Move pivot to the end
int i = low;
for (int j = low; j < high; j++) {
if (nums[j] >= pivotValue) {
swap(nums, i, j);
i++;
}
}
swap(nums, i, high); // Put pivot in its final place
return i;
}
private static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
private static int quickSelect(int[] nums, int low, int high, int k) {
if (low == high) {
return nums[low];
}
int pivotIndex = partition(nums, low, high);
if (k == pivotIndex) {
return nums[k];
} else if (k < pivotIndex) {
return quickSelect(nums, low, pivotIndex - 1, k);
} else {
return quickSelect(nums, pivotIndex + 1, high, k);
}
}
}
```
这个算法的主要思路是不断将数组划分为较小的两部分,直到找到第k小的那个元素。平均时间复杂度为O(n),最坏情况(即输入已经有序)下为O(n^2),但由于引入了随机化,实际运行效率通常很好。
阅读全文
相关推荐
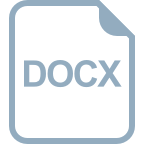
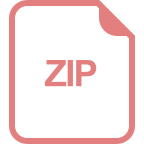
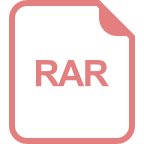















