1.读取指定离线鸢尾花数据集,按照要求完成如下任务. 1.1建立Logistic的3阶多项式; 1.2验证所有训练集,并输出准确率; 1.3绘制最终分类图; 1.4代码关键部分需要加以注释。 写出python代码
时间: 2024-10-18 10:24:53 浏览: 33
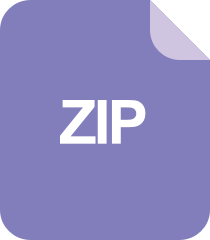
logistic回归分类器鸢尾花_logistic回归_logistic回归分类器_回归分析_鸢尾花数据集_

首先,你需要安装必要的库如`numpy`, `pandas`, `matplotlib`, 和 `sklearn`来进行数据分析、模型构建以及可视化。这里是一个简单的Python代码示例,用于完成上述任务:
```python
# 导入所需的库
import numpy as np
import pandas as pd
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import PolynomialFeatures
import matplotlib.pyplot as plt
# 加载鸢尾花数据集
iris = load_iris()
X = iris.data
y = iris.target
# 对类别标签进行独热编码
encoder = OneHotEncoder(sparse=False)
y_one_hot = encoder.fit_transform(y.reshape(-1, 1))
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y_one_hot, test_size=0.2, random_state=42)
# 创建3阶多项式特征
poly_features = PolynomialFeatures(degree=3)
X_train_poly = poly_features.fit_transform(X_train)
X_test_poly = poly_features.transform(X_test)
# 初始化并训练Logistic回归模型
log_reg = LogisticRegression()
log_reg.fit(X_train_poly, y_train)
# 验证模型并计算训练集准确率
accuracy_train = log_reg.score(X_train_poly, y_train)
print(f"训练集准确率: {accuracy_train * 100:.2f}%")
# 预测并计算测试集准确率
accuracy_test = log_reg.score(X_test_poly, y_test)
print(f"测试集准确率: {accuracy_test * 100:.2f}%")
# 绘制决策边界
plt.figure(figsize=(10, 6))
plot_colors = "rgbcmyk"
for idx, cl in enumerate(np.unique(y)):
plt.scatter(
X[y == cl, 0],
X[y == cl, 1],
marker="o",
color=plot_colors[idx],
label=f"Iris-{cl}",
)
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx, yy = np.meshgrid(np.linspace(x_min, x_max, 500), np.linspace(y_min, y_max, 500))
Z = log_reg.predict(poly_features.transform(np.c_[xx.ravel(), yy.ravel()]))
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.8, cmap=plt.cm.Paired)
plt.xlabel("Sepal length")
plt.ylabel("Sepal width")
plt.legend(loc="upper left")
plt.title("Logistic Regression Decision Boundaries with 3rd Order Polynomial Features")
plt.show()
# 关键代码段注释:
# 1. 使用OneHotEncoder对类别变量进行编码
# 2. 使用PolynomialFeatures创建多项式特征
# 3. 训练和评估Logistic回归模型
# 4. 绘制决策边界区域
```
阅读全文