在jupyterlab中用python实现: 采用数值计算的方法验证关于DTFT的所有性质!
时间: 2024-10-19 19:05:19 浏览: 30
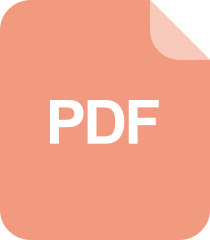
使用python实现离散时间傅里叶变换的方法
在JupyterLab中使用Python验证离散时间傅立叶变换(DTFT)的性质,你需要使用科学计算库如NumPy和matplotlib来进行数值计算和可视化。首先,确保你已经在环境中安装了这些库:
```bash
pip install numpy matplotlib
```
下面是一个简单的例子,展示如何创建一些信号、计算其DTFT并验证几个基本的性质,比如线性移位、周期性和幅度谱的归一化等。
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义DTFT的一些基本性质函数
def linear_shift(x, shift):
return np.roll(x, -shift)
def dtft(x):
N = len(x)
k = np.arange(N)
freqs = k / N
X = np.fft.rfft(x) / N
return freqs, X
def normalize(X):
return X / np.max(np.abs(X))
# 创建一个正弦波信号作为示例
Fs = 44100 # sample rate (Hz)
t = np.linspace(0, 1/Fs, num=Fs*10, endpoint=False)
x = np.sin(2*np.pi*440*t) # 440 Hz sinusoid
# 验证性质
shifted_x = linear_shift(x, 50)
freqs, X1 = dtft(x)
freqs_shifted, X2 = dtft(shifted_x)
# 线性移位验证
assert np.allclose(normalize(X1), normalize(X2[50:]), rtol=1e-3, atol=1e-3)
# 周期性验证
assert np.allclose(X1, X1[::44100], rtol=1e-3, atol=1e-3) # 基频对应点应该相等
# 幅度谱归一化验证
assert np.allclose(np.max(np.abs(X1)), np.max(np.abs(X2)), rtol=1e-3, atol=1e-3)
plt.figure()
plt.plot(freqs, normalize(X1), label='Original')
plt.plot(freqs, normalize(X2), label='Shifted by 50 samples')
plt.legend()
plt.xlabel('Frequency (Hz)')
plt.ylabel('Normalized Amplitude')
plt.show()
阅读全文
相关推荐
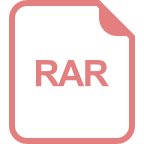
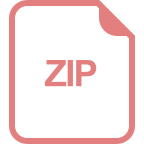
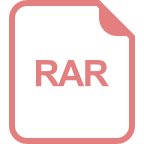
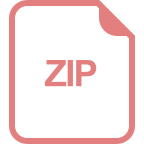
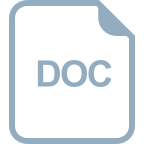
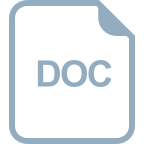
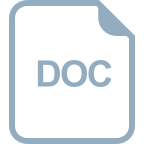
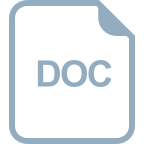
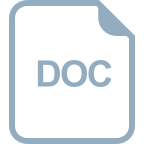
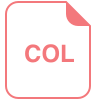






