import torch.optim
时间: 2024-01-31 08:03:18 浏览: 119
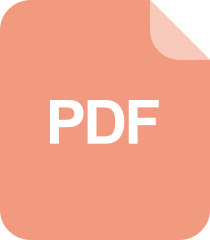
PyTorch上搭建简单神经网络实现回归和分类的示例.pdf
`torch.optim`是PyTorch中用于优化算法的模块。它提供了各种优化器,如随机梯度下降(SGD)、Adam、Adagrad等,用于更新神经网络的参数以最小化损失函数。通过使用`torch.optim`模块,我们可以方便地在训练过程中调整学习率、设置动量、设置权重衰减等。
以下是一个使用`torch.optim`模块的例子:
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 定义模型
model = nn.Linear(10, 2)
# 定义优化器
optimizer = optim.SGD(model.parameters(), lr=0.01, momentum=0.9, weight_decay=1e-5)
# 在训练过程中使用优化器更新模型参数
for epoch in range(100):
# 前向传播和计算损失
loss = ...
# 梯度清零
optimizer.zero_grad()
# 反向传播
loss.backward()
# 更新模型参数
optimizer.step()
```
在上面的例子中,我们使用了`optim.SGD`优化器,它使用随机梯度下降算法来更新模型参数。`lr`参数表示学习率,`momentum`参数表示动量,`weight_decay`参数表示权重衰减。
阅读全文
相关推荐
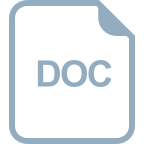
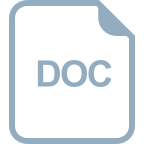
















