React中如何监听其他事件?
时间: 2024-09-25 14:19:24 浏览: 49
在React中,虽然主要关注的是组件间的props传递以及生命周期方法来处理数据变化,但如果你需要监听DOM元素的某些非标准事件(如鼠标滚轮、键盘按键等),你可以通过以下几个步骤来实现:
1. **使用ref**:首先,你需要创建一个`ref`来引用DOM元素,这允许你直接操作DOM。例如:
```jsx
import { useRef } from 'react';
function MyComponent() {
const scrollRef = useRef(null);
function handleScroll(e) {
console.log('Scrolled:', e);
}
return (
<div ref={scrollRef} onWheel={handleScroll}>
...组件内容...
</div>
);
}
```
2. **原生事件处理**:你可以在函数组件里直接绑定事件处理器,如果事件不是由React引发的,你应该手动`event.stopPropagation()`来防止React试图拦截它。
3. **高阶组件(HOC)** 或 `useEffect` hook:有时,你可能希望在组件渲染完成后动态添加事件监听器。这时可以使用`useEffect`或包装组件的方式添加并清理事件监听。
```jsx
import { useEffect } from 'react';
function withEventListener(Component) {
return function EnhancedComponent(props) {
useEffect(() => {
const element = document.getElementById('myElement');
if (element) {
element.addEventListener('customEvent', handleCustomEvent);
// 清理阶段记得移除事件监听
return () => element.removeEventListener('customEvent', handleCustomEvent);
}
}, []);
return <Component {...props} />;
};
}
function MyCustomComponent() {
function handleCustomEvent(event) {
console.log('Custom event:', event);
}
return (
<div id="myElement">
...
</div>
);
}
const EnhancedMyCustomComponent = withEventListener(MyCustomComponent);
```
阅读全文
相关推荐
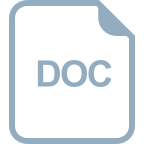
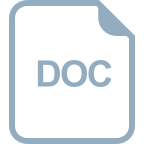
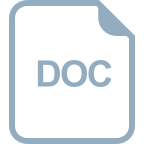


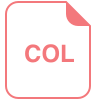











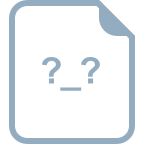