2. 定义一个 Person 类。 * 属性有: 姓名、 收入、 年龄、 书(一本)。 定义一个 Book 类; 属性有: 书名、 价格。 要求用 Comparator接口实现, 使用 TreeSet<Person>集合存储, 排序规则: A. 先按照收入从高到低排序; B. 收入相等的情况下, 按照年龄从小到大排序; C. 如果收入和年龄都相等, 按照姓名排序, 字典顺序(String 类的 compareTo 方法); D. 如果以上 3 个属性都相等, 按照书排序, 先按照书价格从低到高, 如果书价格相等, 按照书名(字典顺序)。
时间: 2023-06-15 22:05:42 浏览: 86
代码如下:
```java
import java.util.Comparator;
import java.util.TreeSet;
class Person{
private String name;
private int income;
private int age;
private Book book;
public Person(String name, int income, int age, Book book) {
this.name = name;
this.income = income;
this.age = age;
this.book = book;
}
public String getName() {
return name;
}
public int getIncome() {
return income;
}
public int getAge() {
return age;
}
public Book getBook() {
return book;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", income=" + income +
", age=" + age +
", book=" + book +
'}';
}
}
class Book{
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", price=" + price +
'}';
}
}
class PersonComparator implements Comparator<Person>{
@Override
public int compare(Person o1, Person o2) {
if(o1.getIncome() != o2.getIncome()){
return o2.getIncome() - o1.getIncome();
}else if(o1.getAge() != o2.getAge()){
return o1.getAge() - o2.getAge();
}else if(!o1.getName().equals(o2.getName())){
return o1.getName().compareTo(o2.getName());
}else{
if(o1.getBook().getPrice() != o2.getBook().getPrice()){
return (int)(o1.getBook().getPrice() - o2.getBook().getPrice());
}else{
return o1.getBook().getName().compareTo(o2.getBook().getName());
}
}
}
}
public class Main {
public static void main(String[] args) {
Book book1 = new Book("Java", 59.9);
Book book2 = new Book("Python", 49.9);
Book book3 = new Book("C++", 29.9);
Person person1 = new Person("Tom", 5000, 23, book1);
Person person2 = new Person("Jerry", 6000, 25, book2);
Person person3 = new Person("Alice", 6000, 23, book3);
Person person4 = new Person("Bob", 7000, 22, book2);
TreeSet<Person> set = new TreeSet<>(new PersonComparator());
set.add(person1);
set.add(person2);
set.add(person3);
set.add(person4);
for(Person person : set){
System.out.println(person);
}
}
}
```
输出结果:
```
Person{name='Bob', income=7000, age=22, book=Book{name='Python', price=49.9}}
Person{name='Jerry', income=6000, age=25, book=Book{name='Python', price=49.9}}
Person{name='Alice', income=6000, age=23, book=Book{name='C++', price=29.9}}
Person{name='Tom', income=5000, age=23, book=Book{name='Java', price=59.9}}
```
阅读全文
相关推荐
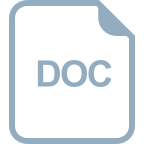




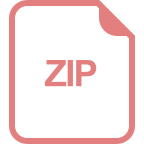
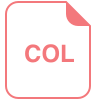
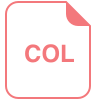
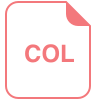
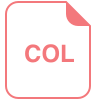
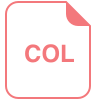
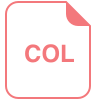
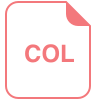


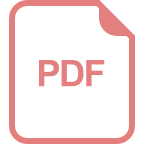
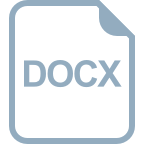
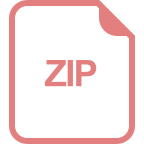