2.定义一个Person类。属性有: 姓名、 收入、 年龄、 书(一本)。 定义一个Book类; 属性有: 书名、 价格。 要求用Comparable接口实现, 使用TreeSet<Person>集合存储, 排序规则: A.先按照收入从高到低排序; B.收入相等的情况下, 按照年龄从小到大排序; C.如果收入和年龄都相等, 按照姓名排序, 字典顺序(String类的compareTo方法); D.如果以上3个属性都相等, 按照书排序, 先按照书价格从低到高, 如果书价格相等, 按照书名(字典顺序)。 3.利用自定义比较器Comparator实现类
时间: 2023-06-15 10:04:47 浏览: 81
2. 实现Person类和Book类,并实现Comparable接口:
```java
public class Person implements Comparable<Person> {
private String name;
private double income;
private int age;
private Book book;
public Person(String name, double income, int age, Book book) {
this.name = name;
this.income = income;
this.age = age;
this.book = book;
}
// Getters and setters
@Override
public int compareTo(Person o) {
int result = Double.compare(o.income, this.income);
if (result == 0) {
result = Integer.compare(this.age, o.age);
if (result == 0) {
result = this.name.compareTo(o.name);
if (result == 0) {
result = this.book.compareTo(o.book);
}
}
}
return result;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", income=" + income +
", age=" + age +
", book=" + book +
'}';
}
}
public class Book implements Comparable<Book> {
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
// Getters and setters
@Override
public int compareTo(Book o) {
int result = Double.compare(this.price, o.price);
if (result == 0) {
result = this.name.compareTo(o.name);
}
return result;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", price=" + price +
'}';
}
}
```
3. 实现Comparator实现类:
```java
public class PersonComparator implements Comparator<Person> {
@Override
public int compare(Person o1, Person o2) {
int result = Double.compare(o2.getIncome(), o1.getIncome());
if (result == 0) {
result = Integer.compare(o1.getAge(), o2.getAge());
if (result == 0) {
result = o1.getName().compareTo(o2.getName());
if (result == 0) {
result = o1.getBook().compareTo(o2.getBook());
}
}
}
return result;
}
}
```
4. 使用TreeSet<Person>集合存储,并按照要求排序:
```java
public class Main {
public static void main(String[] args) {
Book book1 = new Book("Java编程思想", 88.8);
Book book2 = new Book("Effective Java", 66.6);
Book book3 = new Book("Java核心技术", 88.8);
Person person1 = new Person("Alice", 10000, 20, book1);
Person person2 = new Person("Bob", 20000, 25, book2);
Person person3 = new Person("Charlie", 20000, 22, book3);
Person person4 = new Person("David", 30000, 30, book2);
TreeSet<Person> set = new TreeSet<>(new PersonComparator());
set.add(person1);
set.add(person2);
set.add(person3);
set.add(person4);
for (Person person : set) {
System.out.println(person);
}
}
}
```
输出结果为:
```
Person{name='David', income=30000.0, age=30, book=Book{name='Effective Java', price=66.6}}
Person{name='Bob', income=20000.0, age=25, book=Book{name='Effective Java', price=66.6}}
Person{name='Charlie', income=20000.0, age=22, book=Book{name='Java核心技术', price=88.8}}
Person{name='Alice', income=10000.0, age=20, book=Book{name='Java编程思想', price=88.8}}
```
阅读全文
相关推荐
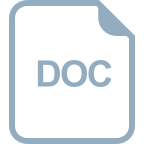
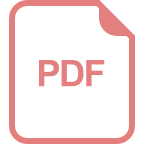
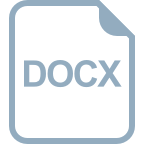
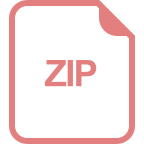
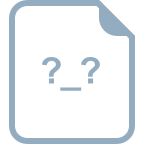
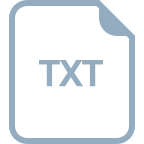
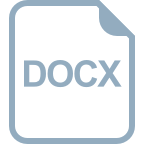
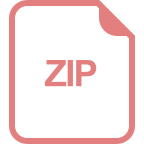
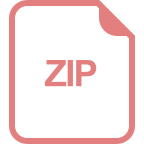
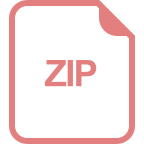
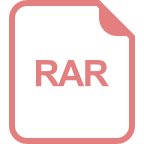
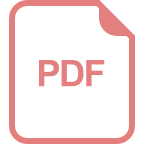
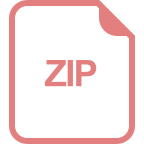
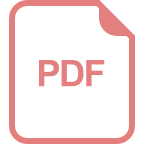