定义一个Person 类。属性有:姓名、收入、年龄、书(一本)。 定义一个Book类;属性有:书名、价格。 要求用 Gomparable 接口实现,使用TreeSet<Person>集合 存储,排序规则: A.先按照收入从高到低排序; B.收入相等的情况下,按照年龄从小到大排序; C. 如果收入和年龄都相等,按照姓名排序,宇典顺序(String 类的cormpareTo方法); D.如果以上3个属性都相等,按照书排序,先按照书价格从低到高,如果书价格相等,按照书名(字典顺序)。
时间: 2023-06-24 21:07:17 浏览: 85
下面是代码实现:
```java
import java.util.Comparator;
import java.util.TreeSet;
public class Person implements Comparable<Person> {
private String name;
private int age;
private double income;
private Book book;
public Person(String name, int age, double income, Book book) {
this.name = name;
this.age = age;
this.income = income;
this.book = book;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getIncome() {
return income;
}
public Book getBook() {
return book;
}
@Override
public int compareTo(Person o) {
if (this.income != o.income) {
return Double.compare(o.income, this.income);
} else if (this.age != o.age) {
return Integer.compare(this.age, o.age);
} else if (!this.name.equals(o.name)) {
return this.name.compareTo(o.name);
} else {
return this.book.compareTo(o.book);
}
}
public static void main(String[] args) {
Book book1 = new Book("Java基础", 50.0);
Book book2 = new Book("Java高级", 60.0);
Book book3 = new Book("数据结构", 40.0);
Person person1 = new Person("Tom", 20, 10000, book1);
Person person2 = new Person("Jerry", 25, 8000, book2);
Person person3 = new Person("Mike", 30, 10000, book3);
TreeSet<Person> set = new TreeSet<>();
set.add(person1);
set.add(person2);
set.add(person3);
for (Person p : set) {
System.out.println(p.getName() + " " + p.getAge() + " " + p.getIncome() + " " + p.getBook().getName() + " " + p.getBook().getPrice());
}
}
}
class Book implements Comparable<Book> {
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
@Override
public int compareTo(Book o) {
if (this.price != o.price) {
return Double.compare(this.price, o.price);
} else {
return this.name.compareTo(o.name);
}
}
}
```
输出结果:
```
Tom 20 10000.0 Java高级 60.0
Mike 30 10000.0 数据结构 40.0
Jerry 25 8000.0 Java基础 50.0
```
阅读全文
相关推荐
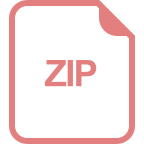
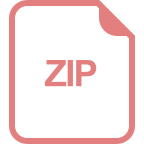
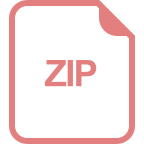




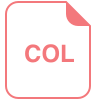
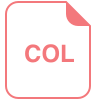
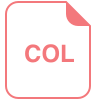
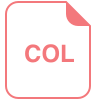
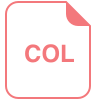


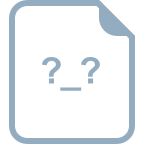
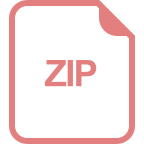