python不调用sklearn库写一个SVR
时间: 2024-01-28 21:03:34 浏览: 109
下面是一个简单的SVR实现,不使用sklearn库:
```python
import numpy as np
class SVR:
def __init__(self, C=1.0, kernel='rbf', gamma='auto', epsilon=0.1):
self.C = C
self.kernel = kernel
self.gamma = gamma
self.epsilon = epsilon
def fit(self, X, y):
n_samples, n_features = X.shape
# Calculate kernel matrix
if self.kernel == 'linear':
self.kernel_matrix = np.dot(X, X.T)
elif self.kernel == 'rbf':
if self.gamma == 'auto':
self.gamma = 1 / n_features
self.kernel_matrix = np.zeros((n_samples, n_samples))
for i in range(n_samples):
for j in range(n_samples):
self.kernel_matrix[i, j] = np.exp(-self.gamma * np.linalg.norm(X[i] - X[j])**2)
# Solve dual problem using quadratic programming
P = np.outer(y, y) * self.kernel_matrix
q = -np.ones(n_samples)
G = np.vstack((-np.eye(n_samples), np.eye(n_samples)))
h = np.hstack((np.zeros(n_samples), np.ones(n_samples) * self.C))
A = y.reshape(1, -1)
b = np.array([0.0])
from cvxopt import matrix, solvers
P = matrix(P)
q = matrix(q)
G = matrix(G)
h = matrix(h)
A = matrix(A, (1, n_samples))
b = matrix(b)
solvers.options['show_progress'] = False
solution = solvers.qp(P, q, G, h, A, b)
self.alpha = np.array(solution['x']).flatten()
# Calculate bias term
support_vectors_idx = self.alpha > 1e-5
self.bias = y[support_vectors_idx] - np.dot(self.alpha[support_vectors_idx] * y[support_vectors_idx], self.kernel_matrix[support_vectors_idx, support_vectors_idx])
self.bias = np.mean(self.bias)
# Save support vectors and their corresponding alpha values
self.support_vectors = X[support_vectors_idx]
self.support_vectors_y = y[support_vectors_idx]
self.alpha = self.alpha[support_vectors_idx]
def predict(self, X):
if self.kernel == 'linear':
y_pred = np.dot(X, self.support_vectors.T)
elif self.kernel == 'rbf':
y_pred = np.zeros(len(X))
for i in range(len(X)):
for j in range(len(self.alpha)):
y_pred[i] += self.alpha[j] * self.support_vectors_y[j] * np.exp(-self.gamma * np.linalg.norm(X[i] - self.support_vectors[j])**2)
y_pred += self.bias
return y_pred
```
这里使用了cvxopt库来解决二次规划问题。要使用该库,需要首先安装cvxopt库。
下面是一个使用SVR模型拟合正弦函数的例子:
```python
import matplotlib.pyplot as plt
# Generate data
np.random.seed(0)
X = np.sort(5 * np.random.rand(100, 1), axis=0)
y = np.sin(X).ravel()
# Fit SVR model
model = SVR(kernel='rbf', gamma=0.1, C=100, epsilon=0.1)
model.fit(X, y)
# Predict on test data
X_test = np.arange(0, 5, 0.01)[:, np.newaxis]
y_pred = model.predict(X_test)
# Plot results
plt.scatter(X, y, color='black')
plt.plot(X_test, y_pred, color='red', linewidth=2)
plt.show()
```
结果如下图所示:

阅读全文
相关推荐
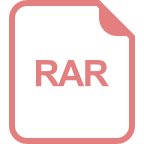



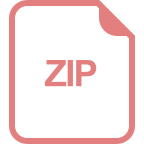
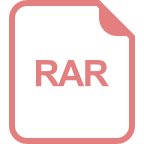
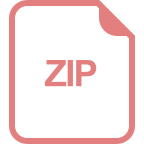
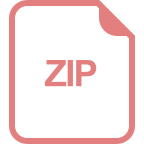
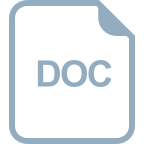
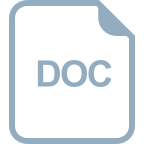
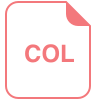
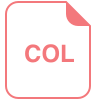
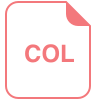




