使用java,查询mysql数据库的学生表(no,name,age,class),打印输出每条记录信息。
时间: 2024-03-01 18:49:46 浏览: 59
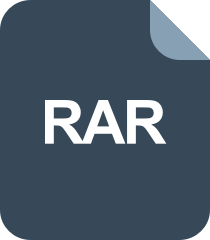
Java写学生成绩系统以及MySQL表格
好的,我可以为您提供代码示例。您可以使用Java中的JDBC API来连接和查询MySQL数据库。以下是一个基本的示例代码,可以查询学生表中的每条记录信息并打印输出:
```java
import java.sql.*;
public class StudentDB {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/STUDENT";
static final String USER = "your_username";
static final String PASS = "your_password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql;
sql = "SELECT no, name, age, class FROM student";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int no = rs.getInt("no");
String name = rs.getString("name");
int age = rs.getInt("age");
String class = rs.getString("class");
System.out.print("No: " + no);
System.out.print(", Name: " + name);
System.out.print(", Age: " + age);
System.out.println(", Class: " + class);
}
rs.close();
stmt.close();
conn.close();
} catch(SQLException se) {
se.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
} finally {
try {
if(stmt!=null)
stmt.close();
} catch(SQLException se2) {
}
try {
if(conn!=null)
conn.close();
} catch(SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
在上面的代码中,您需要将`your_username`和`your_password`替换为您自己的MySQL数据库用户名和密码。同时,您还需要更改`DB_URL`变量以指向您的MySQL数据库的正确位置。如果您的MySQL数据库不在本地计算机上,则需要更改`DB_URL`变量以包括服务器名称和端口号。
执行上面的代码后,它将连接到MySQL数据库并查询学生表中的每条记录信息。然后,它将打印输出每个学生的记录信息。
阅读全文
相关推荐




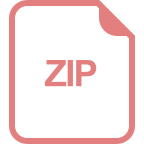










