c++string 类的输入重载
时间: 2023-05-20 08:06:37 浏览: 82
C++中的string类可以通过重载输入运算符(>>)来实现输入操作。下面是一个示例代码:
```
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str;
cout << "请输入一个字符串:";
cin >> str;
cout << "您输入的字符串是:" << str << endl;
return 0;
}
```
在这个示例代码中,我们使用了string类来定义一个字符串变量str,然后通过cin输入运算符来读取用户输入的字符串,并将其存储到str变量中。最后,我们使用cout输出运算符来显示用户输入的字符串。
需要注意的是,输入运算符(>>)默认以空格、制表符或换行符作为输入数据的分隔符,因此如果用户输入的字符串中包含空格等分隔符,那么只会读取到第一个分隔符前的部分作为输入数据。如果需要读取整个字符串,可以使用getline函数来实现。
相关问题
c++中string类运算符重载加法
C++中`string`类已经重载了`+`运算符,因此可以直接使用`+`进行字符串的拼接。例如:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "Hello, ";
string str2 = "world!";
string str3 = str1 + str2;
cout << str3 << endl; // 输出:Hello, world!
return 0;
}
```
如果想要手动实现`+`运算符重载,可以使用以下代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
private:
char* m_data;
public:
MyString(const char* str = nullptr) {
if (str) {
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
} else {
m_data = new char[1];
*m_data = '\0';
}
}
MyString(const MyString& other) {
m_data = new char[strlen(other.m_data) + 1];
strcpy(m_data, other.m_data);
}
~MyString() {
delete[] m_data;
}
MyString operator+(const MyString& other) const {
MyString newStr;
newStr.m_data = new char[strlen(m_data) + strlen(other.m_data) + 1];
strcpy(newStr.m_data, m_data);
strcat(newStr.m_data, other.m_data);
return newStr;
}
friend ostream& operator<<(ostream& os, const MyString& str) {
os << str.m_data;
return os;
}
};
int main() {
MyString str1("Hello, ");
MyString str2("world!");
MyString str3 = str1 + str2;
cout << str3 << endl; // 输出:Hello, world!
return 0;
}
```
注意:以上代码只是一种实现方式,实际应用中应该使用`string`类自带的`+`运算符重载,因为它已经经过了严格的测试和优化。
c++string运算符重载
C++中的string类已经重载了许多运算符,例如+、+=、==、!=、<、<=、>、>=等。我们也可以自己定义运算符重载来实现一些特定的功能。
例如,我们可以重载+运算符来实现两个字符串的拼接:
```c++
string operator+(const string& str1, const string& str2) {
string result = str1;
result += str2;
return result;
}
```
这里我们定义了一个全局函数,接受两个const string&类型的参数,返回一个string类型的结果。在函数内部,我们先将第一个字符串赋值给结果字符串,然后再使用+=运算符将第二个字符串拼接到结果字符串后面。
阅读全文
相关推荐
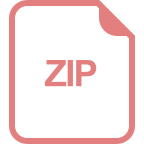
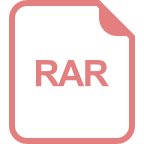
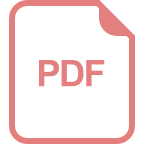













