python中删除Excel中的空行
时间: 2023-07-16 11:13:34 浏览: 101
可以使用Python中的pandas库来实现删除Excel中的空行。具体操作步骤如下:
1. 导入pandas库:
```python
import pandas as pd
```
2. 读取Excel文件:
```python
df = pd.read_excel('your_excel_file.xlsx')
```
3. 删除空行:
```python
df.dropna(how='all', inplace=True)
```
其中,how参数指定了删除的方式,'all'表示只有所有列的值都为NaN时才删除该行。
4. 将处理后的数据重新写入Excel文件:
```python
df.to_excel('new_excel_file.xlsx', index=False)
```
其中,index参数指定是否在输出中包含DataFrame的行索引,默认为True。
完整代码示例:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('your_excel_file.xlsx')
# 删除空行
df.dropna(how='all', inplace=True)
# 将处理后的数据重新写入Excel文件
df.to_excel('new_excel_file.xlsx', index=False)
```
相关问题
python 删除excel空行
你可以使用Python的第三方库`pandas`来删除Excel中的空行。首先,你需要安装`pandas`库,可以使用以下命令安装:
```python
pip install pandas
```
然后,你可以使用以下代码来删除Excel文件中的空行:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('your_excel_file.xlsx')
# 删除空行
df = df.dropna(how='all')
# 保存修改后的Excel文件
df.to_excel('new_excel_file.xlsx', index=False)
```
在这个代码中,`read_excel`函数用于读取Excel文件,并将其存储为一个名为`df`的DataFrame对象。然后,`dropna`函数用于删除包含空值的行,参数`how='all'`表示只删除全部是空值的行。最后,使用`to_excel`函数将修改后的DataFrame保存为一个新的Excel文件。
记得将代码中的`your_excel_file.xlsx`替换为你要处理的实际Excel文件路径,并将`new_excel_file.xlsx`替换为你想要保存的新文件名。
python excel删除空行
你可以使用Python的第三方库`openpyxl`来操作Excel文件,并删除其中的空行。下面是一个示例代码:
```python
from openpyxl import load_workbook
def delete_empty_rows(file_path, sheet_name):
# 加载Excel文件
workbook = load_workbook(file_path)
# 选择工作表
sheet = workbook[sheet_name]
# 逐行遍历工作表
for row in sheet.iter_rows():
# 判断是否为空行
if all(cell.value is None for cell in row):
# 删除空行
sheet.delete_rows(row[0].row, 1)
# 保存修改
workbook.save(file_path)
# 示例用法
delete_empty_rows('example.xlsx', 'Sheet1')
```
以上代码会打开名为'example.xlsx'的Excel文件,并在'Sheet1'工作表中删除所有的空行。你可以根据自己的实际情况修改文件路径和工作表名称。请确保已经安装了`openpyxl`库。
阅读全文
相关推荐
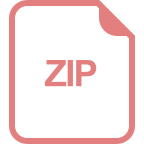
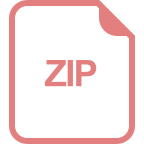
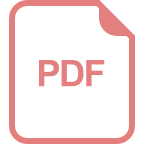













