给出一个实现串的块链存储的 C 语言程序
时间: 2024-10-18 09:23:13 浏览: 20
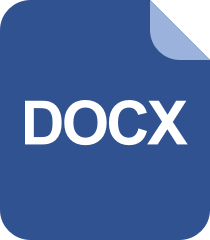
数据结构C语言版 串的块链存储表示和实现.docx
在C语言中,我们可以创建一个简单的串的块链存储结构,以下是一个基础的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义块链节点结构体
typedef struct Node {
char data; // 存储单个字符
struct Node* next; // 指向下一个节点的指针
} Node;
// 定义串的块链结构体
typedef struct StringBlockChain {
Node* head; // 链表头部
int capacity; // 每个块的最大容量
int size; // 当前字符串的实际大小
} StringBlockChain;
// 动态创建一个新的块
Node* create_block(int capacity) {
Node* new_node = (Node*)malloc(sizeof(Node) + capacity - 1);
if (!new_node) return NULL;
new_node->data = '\0'; // 初始化为结束符
new_node->next = NULL;
return new_node;
}
// 插入字符到字符串
void insert_char(StringBlockChain* sbc, char c) {
if (sbc->size >= sbc->capacity * 2) { // 如果已满,扩容
Node* new_head = create_block(sbc->capacity * 2);
if (!new_head) return;
while (sbc->head) {
new_head->next = sbc->head;
sbc->head = new_head;
new_head = sbc->head->next;
}
sbc->capacity *= 2;
}
sbc->head->data = c;
sbc->head->next = sbc->head + 1; // 更新指针
sbc->size++;
}
// 显示字符串
void print_string(StringBlockChain* sbc) {
Node* current = sbc->head;
while (current) {
printf("%c", current->data);
current = current->next;
}
printf("\n");
}
int main() {
StringBlockChain sbc = { .head = create_block(5), .capacity = 5, .size = 0 };
insert_char(&sbc, 'H');
insert_char(&sbc, 'e');
insert_char(&sbc, 'l');
insert_char(&sbc, 'l');
insert_char(&sbc, 'o');
print_string(&sbc);
return 0;
}
```
这个程序首先定义了`Node`和`StringBlockChain`结构,然后实现了创建块、插入字符和打印字符串的功能。注意这只是一个基本版本,实际应用中还需要考虑错误处理和内存释放等细节。
阅读全文
相关推荐
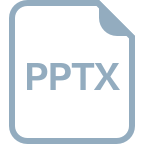
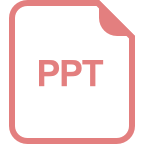
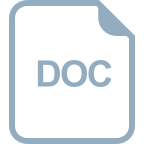
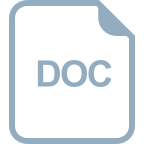
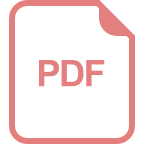
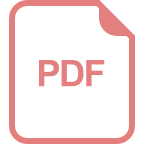
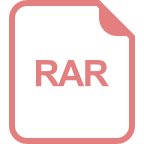
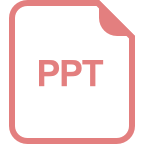
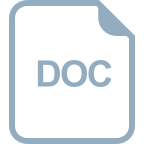
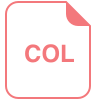
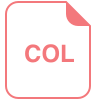
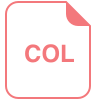
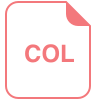
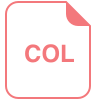


