pytorch如何手动构造RNN模型
时间: 2023-11-16 15:44:09 浏览: 85
首先,需要导入pytorch库:
```python
import torch
import torch.nn as nn
```
然后,我们可以手动定义一个RNN模型,例如一个简单的单层LSTM模型,如下所示:
```python
class LSTM(nn.Module):
"""
单层LSTM模型
"""
def __init__(self, input_size, hidden_size, output_size):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
# 根据输入维度和隐层维度定义权重和偏置
self.i2h = nn.Linear(input_size + hidden_size, hidden_size)
self.i2o = nn.Linear(input_size + hidden_size, output_size)
self.softmax = nn.LogSoftmax(dim=1)
self.lstm = nn.LSTMCell(input_size, hidden_size)
def forward(self, input, hidden):
combined = torch.cat((input, hidden), 1)
hidden = self.i2h(combined)
output = self.i2o(combined)
output = self.softmax(output)
hidden = self.lstm(input, hidden)
return output, hidden
```
这样,我们就手动构造了一个单层LSTM模型。在这个模型中,我们定义了一个nn.Linear层来处理输入和隐层,并使用nn.LSTMCell层作为LSTM的主体。在forward函数中,我们首先将输入和隐层拼接起来,然后通过两个nn.Linear层进行计算,接着对输出进行softmax,并传入LSTMCell层进行计算返回结果。
阅读全文
相关推荐


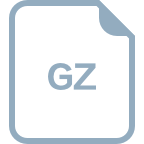













