给出python实现策略自适应和参数自适应的SADE算法的代码
时间: 2024-03-18 15:39:39 浏览: 85
以下是一个简单的Python实现,使用SADE算法实现策略自适应和参数自适应:
```
import random
class SADE:
def __init__(self, pop_size, f, cr, dim, bounds):
self.pop_size = pop_size
self.f = f
self.cr = cr
self.dim = dim
self.bounds = bounds
def optimize(self, max_iter):
# 初始化种群
pop = [[random.uniform(self.bounds[i][0], self.bounds[i][1]) for i in range(self.dim)] for j in range(self.pop_size)]
# 初始化策略和参数
strategy = [0.5] * self.dim
scaling_factor = 0.5
crossover_rate = 0.5
for i in range(max_iter):
for j in range(self.pop_size):
# 根据策略和参数生成新的个体
a, b, c = self.select_samples(pop, j)
mutant = self.mutate(pop, a, b, c, scaling_factor, strategy)
trial = self.crossover(pop[j], mutant, crossover_rate)
# 计算适应度
f_trial = self.f(trial)
if f_trial < self.f(pop[j]):
pop[j] = trial
# 更新策略和参数
strategy, scaling_factor, crossover_rate = self.update_strategy_and_parameters(strategy, scaling_factor, crossover_rate, a, b, c, trial, pop)
best = min(pop, key=self.f)
return best
def select_samples(self, pop, current_index):
# 选择三个不同的个体
a, b, c = random.sample(range(self.pop_size), 3)
while a == current_index:
a = random.randint(0, self.pop_size - 1)
while b == current_index or b == a:
b = random.randint(0, self.pop_size - 1)
while c == current_index or c == a or c == b:
c = random.randint(0, self.pop_size - 1)
return a, b, c
def mutate(self, pop, a, b, c, scaling_factor, strategy):
# 根据策略和参数生成新的个体
mutant = [pop[a][i] + scaling_factor * strategy[i] * (pop[b][i] - pop[c][i]) for i in range(self.dim)]
return mutant
def crossover(self, target, mutant, crossover_rate):
# 生成新的个体
trial = []
for i in range(self.dim):
if random.random() < crossover_rate:
trial.append(mutant[i])
else:
trial.append(target[i])
return trial
def update_strategy_and_parameters(self, strategy, scaling_factor, crossover_rate, a, b, c, trial, pop):
# 计算新的策略和参数
new_strategy = []
for i in range(self.dim):
if random.random() < 0.1:
new_strategy.append(random.uniform(0, 1))
else:
new_strategy.append(strategy[i])
new_scaling_factor = scaling_factor * random.gauss(1, 0.1)
new_crossover_rate = crossover_rate * random.gauss(1, 0.1)
# 判断是否更新策略和参数
if self.f(trial) < self.f(pop[a]) and new_scaling_factor > 0 and new_crossover_rate > 0:
return new_strategy, new_scaling_factor, new_crossover_rate
else:
return strategy, scaling_factor, crossover_rate
```
使用方法:
```
def f(x):
# 目标函数
return x[0] ** 2 + x[1] ** 2
bounds = [(-5, 5), (-5, 5)]
sade = SADE(pop_size=10, f=f, cr=0.5, dim=2, bounds=bounds)
best = sade.optimize(max_iter=100)
print(best)
```
其中,`pop_size`是种群大小,`f`是目标函数,`cr`是交叉率,`dim`是维度,`bounds`是变量的取值范围。在`optimize`方法中,首先初始化种群和策略、参数,然后执行若干代的优化,每次选择三个不同的个体,根据策略和参数生成新的个体,进行交叉和变异,计算适应度,更新种群和策略、参数。最后返回最优个体。
阅读全文
相关推荐
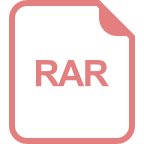
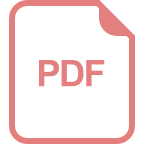
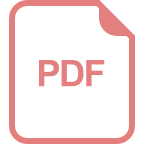
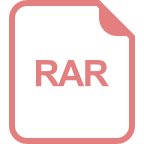
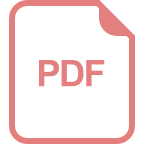
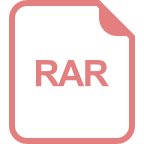
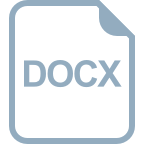
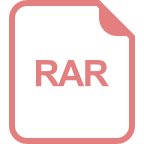
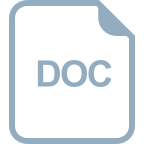
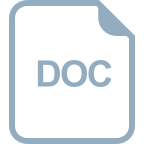
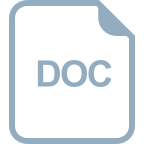



