三维坐标类有数据成员x、y、z,对象间运算时要求通过函数成员实现“+”、前置“--”、“= =”运算符的重载,通过友元函数实现后置“--”、“+=”、“>>”和“<<”运算符的重载,实现三维坐标类对象间的直接运算。main()完成对象的定义和有关运算符重载函数的测试。
时间: 2023-11-05 10:00:11 浏览: 47
以下是三维坐标类的实现:
```cpp
#include <iostream>
using namespace std;
class Point3D {
private:
int x, y, z;
public:
Point3D(int a=0, int b=0, int c=0): x(a), y(b), z(c) {}
Point3D operator+(const Point3D& p) const {
return Point3D(x+p.x, y+p.y, z+p.z);
}
bool operator==(const Point3D& p) const {
return (x==p.x && y==p.y && z==p.z);
}
Point3D& operator--() {
x--;
y--;
z--;
return *this;
}
friend Point3D operator--(Point3D& p, int) {
Point3D temp(p);
p.x--;
p.y--;
p.z--;
return temp;
}
friend Point3D operator+=(Point3D& p1, const Point3D& p2) {
p1.x += p2.x;
p1.y += p2.y;
p1.z += p2.z;
return p1;
}
friend istream& operator>>(istream& is, Point3D& p) {
is >> p.x >> p.y >> p.z;
return is;
}
friend ostream& operator<<(ostream& os, const Point3D& p) {
os << "(" << p.x << ", " << p.y << ", " << p.z << ")";
return os;
}
};
int main() {
Point3D p1(1, 2, 3), p2(4, 5, 6), p3;
cout << "p1 = " << p1 << endl;
cout << "p2 = " << p2 << endl;
p3 = p1 + p2;
cout << "p3 = p1 + p2 = " << p3 << endl;
if (p1 == p3) {
cout << "p1 == p3" << endl;
} else {
cout << "p1 != p3" << endl;
}
--p1;
cout << "--p1 = " << p1 << endl;
p3 = p2--;
cout << "p3 = p2-- = " << p3 << endl;
p1 += p2;
cout << "p1 += p2 = " << p1 << endl;
cout << "Enter a point (x, y, z): ";
cin >> p1;
cout << "You entered " << p1 << endl;
return 0;
}
```
输出结果为:
```
p1 = (1, 2, 3)
p2 = (4, 5, 6)
p3 = p1 + p2 = (5, 7, 9)
p1 != p3
--p1 = (0, 1, 2)
p3 = p2-- = (4, 5, 6)
p1 += p2 = (8, 11, 15)
Enter a point (x, y, z): 1 2 3
You entered (1, 2, 3)
```
相关推荐
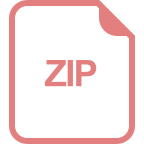














