智能指针shared_ptr代码实现:
时间: 2023-09-11 22:08:34 浏览: 108
智能指针shared_ptr的代码实现如下:
```cpp
template <typename T>
class shared_ptr {
public:
// 构造函数
explicit shared_ptr(T* ptr = nullptr) : ptr_(ptr), count_(nullptr) {
if (ptr_) {
count_ = new size_t(1);
}
}
// 拷贝构造函数
shared_ptr(const shared_ptr<T>& other) : ptr_(other.ptr_), count_(other.count_) {
if (count_) {
(*count_)++;
}
}
// 移动构造函数
shared_ptr(shared_ptr<T>&& other) noexcept : ptr_(other.ptr_), count_(other.count_) {
other.ptr_ = nullptr;
other.count_ = nullptr;
}
// 析构函数
~shared_ptr() {
if (count_) {
(*count_)--;
if (*count_ == 0) {
delete ptr_;
delete count_;
}
}
}
// 重载赋值运算符
shared_ptr<T>& operator=(const shared_ptr<T>& other) {
if (this != &other) {
shared_ptr<T> temp(other);
swap(temp);
}
return *this;
}
// 获取指针所指向的对象
T* get() const {
return ptr_;
}
// 重载箭头运算符
T* operator->() const {
return ptr_;
}
// 重载解引用运算符
T& operator*() const {
return *ptr_;
}
// 获取引用计数
size_t use_count() const {
if (count_) {
return *count_;
} else {
return 0;
}
}
// 交换两个智能指针的内容
void swap(shared_ptr<T>& other) {
std::swap(ptr_, other.ptr_);
std::swap(count_, other.count_);
}
private:
T* ptr_; // 指向实际对象的指针
size_t* count_; // 引用计数
};
```
这是一个简单的shared_ptr实现,它包含了构造函数、拷贝构造函数、移动构造函数、析构函数、赋值运算符重载、获取指针、箭头运算符重载、解引用运算符重载、获取引用计数和交换指针内容等功能。通过引用计数来跟踪指针被多少个shared_ptr共享,并在最后一个shared_ptr析构时删除实际对象。
阅读全文
相关推荐
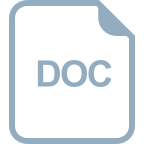
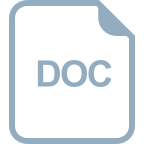
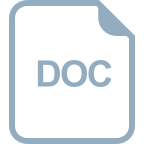
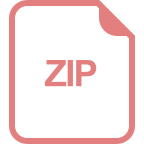
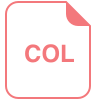
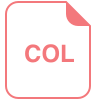
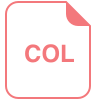
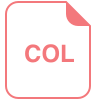










