sem_destroy
时间: 2023-08-30 14:10:55 浏览: 94
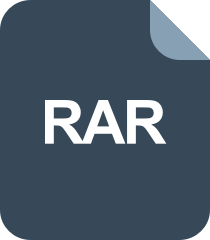
destroy
sem_destroy is a function in the C programming language that is used to destroy a named or unnamed semaphore.
Syntax:
```
#include <semaphore.h>
int sem_destroy(sem_t *sem);
```
Parameters:
- `sem`: Pointer to the semaphore that needs to be destroyed.
Return Value:
The sem_destroy function returns 0 on success, and -1 on failure.
Description:
The sem_destroy function is used to destroy a semaphore that was previously created using the sem_init or sem_open function. Once a semaphore has been destroyed, all threads waiting on it will be unblocked and any resources associated with it will be released.
Note:
- Before calling sem_destroy, all threads waiting on the semaphore must be unblocked.
- Once a semaphore has been destroyed, it cannot be used again until it is re-initialized using sem_init or sem_open.
Example:
```
#include <stdio.h>
#include <semaphore.h>
#include <pthread.h>
sem_t semaphore;
void* myThread(void* arg){
sem_wait(&semaphore);
printf("Thread is waiting on semaphore\n");
sem_post(&semaphore);
printf("Thread has finished waiting on semaphore\n");
return NULL;
}
int main(){
sem_init(&semaphore, 0, 1);
pthread_t threadID;
pthread_create(&threadID, NULL, &myThread, NULL);
pthread_join(threadID, NULL);
sem_destroy(&semaphore);
printf("Semaphore has been destroyed\n");
return 0;
}
```
In the above example, the sem_destroy function is called to destroy the semaphore after the thread has finished waiting on it.
阅读全文
相关推荐
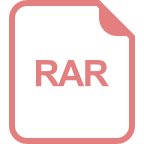
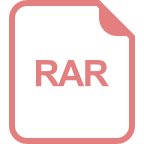















