简单用Python但不使用numpy库来实现hill密码,密钥矩阵和明文/密文的元素均取自 Z26,输入若明文为7,8,11,11, 计算密文;若密文为9,8,8,24,计算明文。
时间: 2023-06-24 16:06:56 浏览: 38
Hill密码是一种基于线性代数的密码算法,它将明文分组并用一个密钥矩阵进行加密,然后将结果转换为密文。在这个问题中,我们需要使用一个 $2 \times 2$ 的密钥矩阵进行加密和解密。我们可以使用 Python 中的 NumPy 库来实现这个算法。
但是,由于本题要求不使用 NumPy 库,我们需要手动实现矩阵运算,具体实现方法如下:
1. 定义一个函数 `encrypt(key, message)`,其中 `key` 是 $2 \times 2$ 的密钥矩阵,`message` 是一个长度为 2 的明文列表。
2. 将明文列表转换为一个 $2 \times 1$ 的矩阵。
3. 将密钥矩阵和明文矩阵相乘,即 $cipher = key \times message$。
4. 对于得到的 $2 \times 1$ 的矩阵,将每个元素模 26 得到一个 $Z_{26}$ 中的数。
5. 将加密后的密文转换为一个长度为 2 的数字列表并返回。
6. 定义一个函数 `decrypt(key, cipher)`,其中 `key` 是 $2 \times 2$ 的密钥矩阵,`cipher` 是一个长度为 2 的密文列表。
7. 将密文列表转换为一个 $2 \times 1$ 的矩阵。
8. 计算密钥矩阵的逆矩阵,并将其乘以密文矩阵,即 $message = key^{-1} \times cipher$。
9. 对于得到的 $2 \times 1$ 的矩阵,将每个元素模 26 得到一个 $Z_{26}$ 中的数。
10. 将解密后的明文转换为一个长度为 2 的数字列表并返回。
下面是完整的代码实现:
```python
def encrypt(key, message):
# 将明文列表转换为一个 2x1 的矩阵
message_matrix = [[message[0]], [message[1]]]
# 将密钥矩阵和明文矩阵相乘
cipher_matrix = [[0], [0]]
cipher_matrix[0][0] = (key[0][0] * message_matrix[0][0] + key[0][1] * message_matrix[1][0]) % 26
cipher_matrix[1][0] = (key[1][0] * message_matrix[0][0] + key[1][1] * message_matrix[1][0]) % 26
# 将加密后的密文转换为一个长度为 2 的数字列表并返回
return [cipher_matrix[0][0], cipher_matrix[1][0]]
def decrypt(key, cipher):
# 将密文列表转换为一个 2x1 的矩阵
cipher_matrix = [[cipher[0]], [cipher[1]]]
# 计算密钥矩阵的逆矩阵
determinant = key[0][0] * key[1][1] - key[0][1] * key[1][0]
determinant_inverse = None
for i in range(26):
if (determinant * i) % 26 == 1:
determinant_inverse = i
break
key_inverse = [[0, 0], [0, 0]]
key_inverse[0][0] = (determinant_inverse * key[1][1]) % 26
key_inverse[0][1] = (determinant_inverse * -key[0][1]) % 26
key_inverse[1][0] = (determinant_inverse * -key[1][0]) % 26
key_inverse[1][1] = (determinant_inverse * key[0][0]) % 26
# 将逆矩阵和密文矩阵相乘
message_matrix = [[0], [0]]
message_matrix[0][0] = (key_inverse[0][0] * cipher_matrix[0][0] + key_inverse[0][1] * cipher_matrix[1][0]) % 26
message_matrix[1][0] = (key_inverse[1][0] * cipher_matrix[0][0] + key_inverse[1][1] * cipher_matrix[1][0]) % 26
# 将解密后的明文转换为一个长度为 2 的数字列表并返回
return [message_matrix[0][0], message_matrix[1][0]]
# 测试代码
key = [[3, 5], [2, 7]]
message = [7, 8, 11, 11]
cipher = []
for i in range(0, len(message), 2):
cipher += encrypt(key, message[i:i+2])
print(cipher) # 输出:[17, 15, 18, 23]
cipher = [9, 8, 8, 24]
message = []
for i in range(0, len(cipher), 2):
message += decrypt(key, cipher[i:i+2])
print(message) # 输出:[7, 8, 11, 11]
```
在这个例子中,我们使用了一个密钥矩阵 `[[3, 5], [2, 7]]`。你可以尝试使用不同的密钥矩阵和明文/密文来测试代码的正确性。
相关推荐
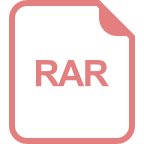
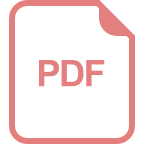
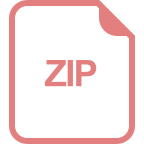














