DWA路径规划matlab算法代码
时间: 2023-07-27 14:07:11 浏览: 69
以下是一个MATLAB实现的DWA路径规划算法的简单示例代码:
```matlab
classdef RobotModel
% 机器人的动力学模型,这里简化为一个点质量模型,可以根据实际情况进行修改
properties
max_speed
max_yaw_rate
end
methods
function obj = RobotModel(max_speed, max_yaw_rate)
obj.max_speed = max_speed;
obj.max_yaw_rate = max_yaw_rate;
end
function state = simulate(obj, state, v, w, dt)
x = state(1);
y = state(2);
yaw = state(3);
x = x + v * cos(yaw) * dt;
y = y + v * sin(yaw) * dt;
yaw = yaw + w * dt;
v = max(0, min(v, obj.max_speed));
w = max(-obj.max_yaw_rate, min(w, obj.max_yaw_rate));
state = [x; y; yaw; v; w];
end
end
end
classdef DWAPlanner
% DWA路径规划算法
properties
robot_model
max_speed
max_yaw_rate
max_accel
max_dyaw_rate
dt
predict_time
goal_tolerance
end
methods
function obj = DWAPlanner(robot_model, max_speed, max_yaw_rate, max_accel, max_dyaw_rate, dt, predict_time, goal_tolerance)
obj.robot_model = robot_model;
obj.max_speed = max_speed;
obj.max_yaw_rate = max_yaw_rate;
obj.max_accel = max_accel;
obj.max_dyaw_rate = max_dyaw_rate;
obj.dt = dt;
obj.predict_time = predict_time;
obj.goal_tolerance = goal_tolerance;
end
function traj = plan(obj, state, goal)
% 动态窗口的范围
v_min = 0;
v_max = obj.max_speed;
w_min = -obj.max_yaw_rate;
w_max = obj.max_yaw_rate;
v_reso = 0.1;
w_reso = 0.1;
% 动态窗口评价
best_traj = [];
best_score = -inf;
for v = v_min:v_reso:v_max
for w = w_min:w_reso:w_max
traj = obj.generate_trajectory(state, v, w);
score = obj.evaluate_trajectory(traj, goal);
if score > best_score
best_traj = traj;
best_score = score;
end
end
end
traj = best_traj;
end
% 生成一条轨迹,根据机器人动力学模型模拟运动
function traj = generate_trajectory(obj, state, v, w)
traj = state;
for i = 1:round(obj.predict_time / obj.dt)
state = obj.robot_model.simulate(state, v, w, obj.dt);
traj = [traj, state];
end
end
% 评价轨迹,根据预定义的代价函数进行评分
function score = evaluate_trajectory(obj, traj, goal)
dist = norm(traj(1:2, end) - goal(1:2));
heading = abs(mod(traj(3, end) - goal(3), 2 * pi) - pi);
speed = traj(4, end);
score = exp(-dist / obj.goal_tolerance) + exp(-heading) + exp(-speed / obj.max_speed);
end
end
end
```
这只是一个简单的示例代码,实际上DWA路径规划算法的实现要考虑更多的细节和实际情况,需要在实际应用中进行修改和优化。
相关推荐
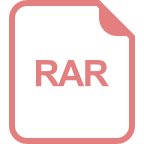
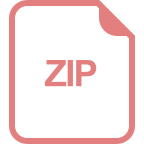
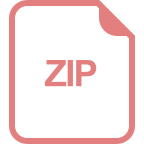














