softmax回归python实现
时间: 2023-07-18 22:15:39 浏览: 112
好的,我来为您讲解一下 softmax 回归的 Python 实现。
首先,我们需要导入一些必要的库,如下所示:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
```
接下来,我们可以使用 make_classification 函数生成一些数据,以便后续模型训练和测试:
```python
X, y = make_classification(n_samples=1000, n_features=2, n_informative=2, n_redundant=0, n_classes=3, random_state=42)
```
然后,我们将数据集分为训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们需要定义 softmax 函数和梯度下降函数。softmax 函数的定义如下:
```python
def softmax(z):
exp_z = np.exp(z - np.max(z, axis=1, keepdims=True))
return exp_z / np.sum(exp_z, axis=1, keepdims=True)
```
梯度下降函数的定义如下:
```python
def gradient_descent(X, y, learning_rate=0.1, num_iterations=1000):
num_classes = len(np.unique(y))
num_features = X.shape[1]
theta = np.zeros((num_features, num_classes))
for i in range(num_iterations):
z = np.dot(X, theta)
y_pred = softmax(z)
gradient = np.dot(X.T, (y_pred - (y == np.arange(num_classes))))
theta -= learning_rate * gradient
return theta
```
最后,我们可以使用训练集进行模型训练,并使用测试集进行模型测试:
```python
theta = gradient_descent(X_train, y_train)
z_test = np.dot(X_test, theta)
y_pred = np.argmax(softmax(z_test), axis=1)
accuracy = np.mean(y_pred == y_test)
print('Accuracy:', accuracy)
```
以上就是 softmax 回归的 Python 实现过程。希望能对您有所帮助。
阅读全文
相关推荐
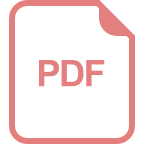
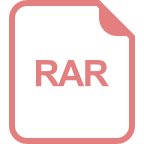















