用c++实现凯撒密码
时间: 2024-08-30 14:02:08 浏览: 30
凯撒密码是一种简单的替换加密技术,通过将明文中的每个字符按照固定的偏移量向字母表移动来加密信息。在C++中实现凯撒密码,你可以创建一个函数,接受明文、偏移量和字符集作为输入。下面是一个基本的实现示例:
```cpp
#include <iostream>
#include <string>
char shift(char c, int offset, bool is_uppercase) {
if (is_uppercase) {
return static_cast<char>((c - 'A' + offset) % 26 + 'A');
} else {
return static_cast<char>((c - 'a' + offset) % 26 + 'a');
}
}
std::string caesar_cipher(const std::string& plaintext, int shift_amount) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
ciphertext += shift(c, shift_amount, isupper(c));
} else {
ciphertext += c; // 非字母字符不变
}
}
return ciphertext;
}
int main() {
std::string plaintext = "Hello, World!";
int shift = 3; // 示例偏移量
std::string ciphertext = caesar_cipher(plaintext, shift);
std::cout << "原始文本: " << plaintext << std::endl;
std::cout << "加密后的文本: " << ciphertext << std::endl;
return 0;
}
```
在这个例子中,`shift` 函数负责处理单个字符的加密,`caesar_cipher` 函数则遍历整个字符串并应用这个函数。注意,只有字母会被加密,其他字符保持不变。
相关推荐
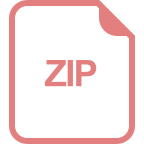
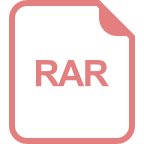
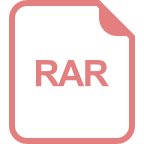
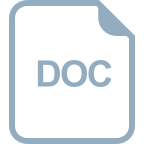
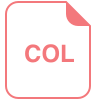
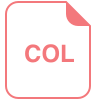
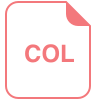
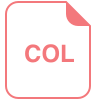
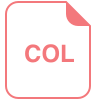








