如何用for模拟一个超市购物系统
时间: 2024-09-29 21:10:06 浏览: 12
要使用 `for` 循环模拟一个简单的超市购物系统,我们可以创建一个程序,让用户选择商品、添加到购物车、查看购物车并结账。以下是一个基本的伪代码示例:
```cpp
#include <iostream>
#include <map> // 使用 map 存储商品信息
// 商品结构体或类
struct Product {
std::string name;
double price;
};
// 商品库存(这里只是一个简单的键值对存储)
std::map<std::string, Product> products = {
{"Apple", { "Apple", 0.5 }}, // 示例商品:苹果,价格0.5元
{"Banana", { "Banana", 0.3 }},
{"Orange", { "Orange", 0.6 }}
};
// 用户操作
void displayProducts() {
for (const auto &product : products) {
std::cout << product.first << ": $" << product.second.price << "\n";
}
}
void addToCart(const std::string &name, int quantity) {
if (products.find(name) != products.end()) {
products[name].price *= quantity; // 假设每个单位打折
std::cout << "Added " << quantity << " " << name << " to cart.\n";
} else {
std::cout << "Product not found.\n";
}
}
double calculateTotal() {
double total = 0;
for (const auto &item : products) {
total += item.second.price;
}
return total;
}
int main() {
bool continueShopping = true;
while (continueShopping) {
displayProducts();
std::string productName;
int quantity;
std::cout << "Enter a product name to add to cart or 'quit' to exit: ";
std::cin >> productName;
if (productName == "quit") {
continueShopping = false;
} else {
std::cout << "How many " << productName << "? ";
std::cin >> quantity;
addToCart(productName, quantity);
}
std::cout << "Your current total is: $" << calculateTotal() << "\n";
}
std::cout << "Thank you for your purchase!\n";
return 0;
}
```
这个程序定义了一个商品库存(这里使用了 `std::map`),展示了所有可用的商品,让用户选择购买,然后计算购物车总价。如果用户输入“quit”,则退出购物过程。
相关推荐
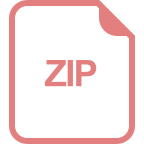
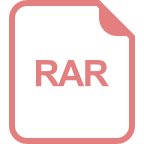
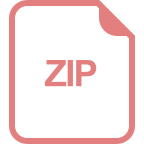















