建立单链表用头插法用c++
时间: 2024-09-25 08:05:35 浏览: 31
在C++中,使用头插法(也称为前插入法)创建单链表通常涉及以下几个步骤:
1. **定义节点结构**:
```cpp
struct Node {
int data; // 节点存储的数据
Node* next; // 指向下一个节点的指针
Node(int value = 0) : data(value), next(nullptr) {} // 构造函数,初始化数据和next为nullptr
};
```
2. **创建头结点**:
```cpp
Node* head = nullptr; // 初始时链表为空,头结点指向nullptr
```
3. **插入操作**:
- 定义一个用于插入新节点到链表头部的函数:
```cpp
void insertAtStart(Node*& head, int value) {
Node* newNode = new Node(value); // 创建新节点
newNode->next = head; // 新节点的next指向前一个头结点
head = newNode; // 更新头结点为新节点
}
```
4. **示例**:
```cpp
int main() {
// 插入元素
insertAtStart(head, 5);
insertAtStart(head, 3);
insertAtStart(head, 7);
// 输出链表(这里只是一个示例,实际需要配合循环遍历)
std::cout << "Head of the list: ";
printList(head); // 自定义的打印链表函数
return 0;
}
// 辅助函数,用于打印链表
void printList(Node* node) {
while (node != nullptr) {
std::cout << node->data << " -> ";
node = node->next;
}
std::cout << "nullptr" << std::endl;
}
```
阅读全文
相关推荐
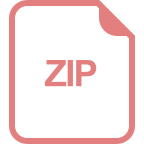
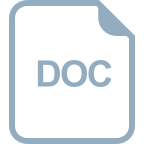














