hog+svm python
时间: 2023-07-08 09:34:54 浏览: 60
HOG (Histogram of Oriented Gradients) and SVM (Support Vector Machine) are commonly used algorithms in computer vision for object detection and classification tasks.
To use HOG and SVM together in Python, you can follow these steps:
1. Collect training data and extract HOG features from the images.
2. Train an SVM classifier on the extracted HOG features.
3. Collect testing data and extract HOG features from the images.
4. Use the trained SVM classifier to predict the class labels of the testing data.
Here is some sample code to get you started:
```
import cv2
import numpy as np
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
# Load the training data and labels
train_data = np.load('train_data.npy')
train_labels = np.load('train_labels.npy')
# Extract HOG features from the training data
hog = cv2.HOGDescriptor()
train_hog = []
for img in train_data:
hog_features = hog.compute(img)
train_hog.append(hog_features)
# Train an SVM classifier on the HOG features
svm = SVC(kernel='linear', C=1.0)
svm.fit(train_hog, train_labels)
# Load the testing data and labels
test_data = np.load('test_data.npy')
test_labels = np.load('test_labels.npy')
# Extract HOG features from the testing data
test_hog = []
for img in test_data:
hog_features = hog.compute(img)
test_hog.append(hog_features)
# Use the trained SVM classifier to predict the class labels of the testing data
pred_labels = svm.predict(test_hog)
# Calculate the accuracy of the classifier
accuracy = accuracy_score(test_labels, pred_labels)
print('Accuracy:', accuracy)
```
Note that this is just a basic example and you may need to tune the parameters of the HOG and SVM algorithms to achieve better performance on your specific task.
相关推荐
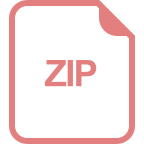
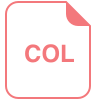
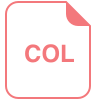
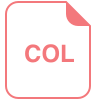











