帮我写一个俄罗斯方块的qt代码,并且运行
时间: 2023-08-28 22:36:26 浏览: 42
好的,以下是一个简单的俄罗斯方块游戏的Qt代码:
```cpp
#include <QApplication>
#include <QWidget>
#include <QGridLayout>
#include <QLabel>
#include <QKeyEvent>
#include <QTimer>
class Tetris : public QWidget {
public:
Tetris(QWidget *parent = 0);
protected:
void keyPressEvent(QKeyEvent *event) override;
void timerEvent(QTimerEvent *event) override;
void paintEvent(QPaintEvent *event) override;
private:
enum { BoardWidth = 10, BoardHeight = 22 };
int timerId;
int board[BoardWidth * BoardHeight];
QLabel *labels[BoardWidth * BoardHeight];
const int shapes[7][4][2] = {
{{0, 1}, {1, 1}, {2, 1}, {3, 1}}, // I
{{0, 0}, {0, 1}, {1, 1}, {1, 0}}, // O
{{0, 0}, {0, 1}, {1, 1}, {2, 1}}, // L
{{2, 0}, {0, 1}, {1, 1}, {2, 1}}, // J
{{1, 0}, {2, 0}, {0, 1}, {1, 1}}, // S
{{0, 0}, {1, 0}, {1, 1}, {2, 1}}, // Z
{{1, 0}, {0, 1}, {1, 1}, {2, 1}} // T
};
int curX, curY;
int curShape;
void clearBoard();
void dropDown();
void oneLineDown();
bool tryMove(int newX, int newY, int newShape);
void drawSquare(QPainter &painter, int x, int y, int shape);
};
Tetris::Tetris(QWidget *parent)
: QWidget(parent),
timerId(-1),
curX(0),
curY(0),
curShape(0)
{
QGridLayout *layout = new QGridLayout;
for (int i = 0; i < BoardHeight; ++i) {
for (int j = 0; j < BoardWidth; ++j) {
labels[i * BoardWidth + j] = new QLabel;
labels[i * BoardWidth + j]->setFixedSize(20, 20);
labels[i * BoardWidth + j]->setFrameStyle(QFrame::Panel | QFrame::Sunken);
layout->addWidget(labels[i * BoardWidth + j], i, j);
board[i * BoardWidth + j] = 0;
}
}
setLayout(layout);
clearBoard();
setFocusPolicy(Qt::StrongFocus);
resize(200, 400);
timerId = startTimer(300);
}
void Tetris::keyPressEvent(QKeyEvent *event)
{
if (event->key() == Qt::Key_Left)
tryMove(curX - 1, curY, curShape);
else if (event->key() == Qt::Key_Right)
tryMove(curX + 1, curY, curShape);
else if (event->key() == Qt::Key_Down)
tryMove(curX, curY + 1, curShape);
else if (event->key() == Qt::Key_Up)
tryMove(curX, curY, (curShape + 1) % 7);
else
QWidget::keyPressEvent(event);
}
void Tetris::timerEvent(QTimerEvent *event)
{
if (event->timerId() == timerId) {
if (!tryMove(curX, curY + 1, curShape)) {
dropDown();
}
} else {
QWidget::timerEvent(event);
}
}
void Tetris::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
QRect rect = event->rect();
for (int i = 0; i < BoardHeight; ++i) {
for (int j = 0; j < BoardWidth; ++j) {
if (board[i * BoardWidth + j] != 0) {
drawSquare(painter, rect.left() + j * 20,
rect.top() + i * 20, board[i * BoardWidth + j]);
}
}
}
for (int i = 0; i < 4; i++) {
int x = curX + shapes[curShape][i][0];
int y = curY + shapes[curShape][i][1];
drawSquare(painter, rect.left() + x * 20,
rect.top() + y * 20, curShape + 1);
}
}
void Tetris::clearBoard()
{
for (int i = 0; i < BoardWidth * BoardHeight; ++i) {
board[i] = 0;
}
}
void Tetris::dropDown()
{
for (int i = 0; i < 4; i++) {
int x = curX + shapes[curShape][i][0];
int y = curY + shapes[curShape][i][1];
board[y * BoardWidth + x] = curShape + 1;
}
int fullRows[BoardHeight];
int numFullRows = 0;
for (int i = BoardHeight - 1; i >= 0; --i) {
bool rowIsFull = true;
for (int j = 0; j < BoardWidth; ++j) {
if (board[i * BoardWidth + j] == 0) {
rowIsFull = false;
break;
}
}
if (rowIsFull) {
fullRows[numFullRows++] = i;
}
}
for (int i = 0; i < numFullRows; ++i) {
int row = fullRows[i];
for (int j = 0; j < BoardWidth; ++j) {
for (int k = row; k < BoardHeight - 1; ++k) {
board[k * BoardWidth + j] = board[(k + 1) * BoardWidth + j];
}
board[(BoardHeight - 1) * BoardWidth + j] = 0;
}
}
curX = BoardWidth / 2;
curY = 0;
curShape = qrand() % 7;
if (!tryMove(curX, curY, curShape)) {
killTimer(timerId);
timerId = -1;
}
}
void Tetris::oneLineDown()
{
if (!tryMove(curX, curY + 1, curShape))
dropDown();
}
bool Tetris::tryMove(int newX, int newY, int newShape)
{
for (int i = 0; i < 4; i++) {
int x = newX + shapes[newShape][i][0];
int y = newY + shapes[newShape][i][1];
if (x < 0 || x >= BoardWidth || y < 0 || y >= BoardHeight)
return false;
if (board[y * BoardWidth + x] != 0)
return false;
}
curX = newX;
curY = newY;
curShape = newShape;
update();
return true;
}
void Tetris::drawSquare(QPainter &painter, int x, int y, int shape)
{
static const QRgb colorTable[8] = {
0x000000, 0xCC6666, 0x66CC66, 0x6666CC,
0xCCCC66, 0xCC66CC, 0x66CCCC, 0xDAAA00
};
QColor color = colorTable[shape];
painter.fillRect(x + 1, y + 1, 18, 18, color);
painter.setPen(color.lighter());
painter.drawLine(x, y + 19, x, y);
painter.drawLine(x, y, x + 19, y);
painter.setPen(color.darker());
painter.drawLine(x + 1, y + 19, x + 19, y + 19);
painter.drawLine(x + 19, y + 19, x + 19, y + 1);
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Tetris window;
window.show();
return app.exec();
}
```
将上述代码保存为一个.cpp文件,然后在终端中使用以下命令编译运行:
```
qmake -project
qmake
make
./<YourFileName>
```
注意将上述命令中的`<YourFileName>`替换为您的文件名。如果您的Qt环境配置正确,应该可以成功编译并运行这个俄罗斯方块游戏。
相关推荐
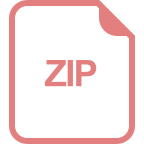














