帮我写一个python代码的俄罗斯方块
时间: 2023-12-31 22:06:56 浏览: 61
抱歉,作为AI语言模型,我无法编写和执行代码。但是,我可以提供一个基本的俄罗斯方块游戏实现,其中包括游戏板、方块、移动和旋转等功能。
```python
import pygame
import random
# 游戏板大小
board_width = 10
board_height = 20
# 方块大小
block_size = 30
# 方块形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 方块颜色
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 游戏板类
class Board:
def __init__(self):
self.board = [[0] * board_width for _ in range(board_height)]
# 判断是否可以放置方块
def is_valid(self, shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if y + i < 0 or x + j < 0 or y + i >= board_height or x + j >= board_width or self.board[y + i][x + j] != 0:
return False
return True
# 放置方块
def place_block(self, shape, x, y, color):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
self.board[y + i][x + j] = color
# 消除已满行
def clear_rows(self):
rows_cleared = 0
for i in range(board_height):
if all(self.board[i]):
self.board.pop(i)
self.board.insert(0, [0] * board_width)
rows_cleared += 1
return rows_cleared
# 判断游戏是否结束
def is_game_over(self):
return any(self.board[0])
# 方块类
class Block:
def __init__(self):
self.shape = random.choice(shapes)
self.color = random.randint(1, len(colors) - 1)
self.x = board_width // 2 - len(self.shape[0]) // 2
self.y = -len(self.shape)
# 移动方块
def move(self, dx, dy, board):
if board.is_valid(self.shape, self.x + dx, self.y + dy):
self.x += dx
self.y += dy
return True
return False
# 旋转方块
def rotate(self, board):
new_shape = []
for i in range(len(self.shape[0])):
row = []
for j in range(len(self.shape)):
row.append(self.shape[len(self.shape) - j - 1][i])
new_shape.append(row)
if board.is_valid(new_shape, self.x, self.y):
self.shape = new_shape
return True
return False
# 游戏类
class Game:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((board_width * block_size, board_height * block_size))
pygame.display.set_caption('俄罗斯方块')
self.clock = pygame.time.Clock()
# 初始化游戏板和方块
self.board = Board()
self.block = Block()
# 绘制游戏板
def draw_board(self):
for i in range(board_height):
for j in range(board_width):
pygame.draw.rect(self.screen, colors[self.board.board[i][j]], (j * block_size, i * block_size, block_size, block_size), 0)
# 绘制方块
def draw_block(self):
for i in range(len(self.block.shape)):
for j in range(len(self.block.shape[i])):
if self.block.shape[i][j] != 0:
pygame.draw.rect(self.screen, colors[self.block.color], ((self.block.x + j) * block_size, (self.block.y + i) * block_size, block_size, block_size), 0)
# 运行游戏
def run(self):
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.block.move(-1, 0, self.board)
elif event.key == pygame.K_RIGHT:
self.block.move(1, 0, self.board)
elif event.key == pygame.K_DOWN:
self.block.move(0, 1, self.board)
elif event.key == pygame.K_UP:
self.block.rotate(self.board)
# 移动方块
if not self.block.move(0, 1, self.board):
self.board.place_block(self.block.shape, self.block.x, self.block.y, self.block.color)
rows_cleared = self.board.clear_rows()
if rows_cleared > 0:
score += rows_cleared * 10
self.block = Block()
# 判断游戏是否结束
if self.board.is_game_over():
pygame.quit()
exit()
# 绘制游戏界面
self.screen.fill((255, 255, 255))
self.draw_board()
self.draw_block()
pygame.display.update()
# 控制游戏速度
self.clock.tick(30)
# 运行游戏
if __name__ == '__main__':
game = Game()
game.run()
```
这个实现可能不是最优的,但可以作为一个基本的参考。
阅读全文
相关推荐
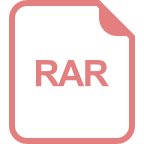
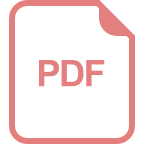















