帮我python写俄罗斯方块
时间: 2023-07-25 19:22:42 浏览: 79
好的,以下是一个简单的Python实现俄罗斯方块游戏的示例代码,你可以根据自己的需要进行修改和完善。
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 定义游戏窗口的大小
WINDOW_WIDTH = 300
WINDOW_HEIGHT = 600
# 定义方块的大小和形状
BLOCK_SIZE = 30
SHAPES = [
[(0, 0), (1, 0), (2, 0), (3, 0)], # I 形
[(0, 0), (1, 0), (0, 1), (1, 1)], # O 形
[(0, 1), (1, 1), (2, 1), (2, 0)], # L 形
[(0, 0), (1, 0), (2, 0), (2, 1)], # J 形
[(0, 1), (1, 1), (1, 0), (2, 0)], # S 形
[(0, 0), (1, 0), (1, 1), (2, 1)], # Z 形
[(0, 1), (1, 1), (1, 0), (2, 1)] # T 形
]
# 定义方块的颜色
COLORS = [
(0, 255, 255), # 青色
(255, 255, 0), # 黄色
(255, 0, 0), # 红色
(0, 0, 255), # 蓝色
(0, 255, 0), # 绿色
(255, 0, 255), # 紫色
(255, 128, 0) # 橙色
]
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 定义字体
font = pygame.font.SysFont(None, 30)
# 定义游戏得分
score = 0
# 定义方块类
class Block:
def __init__(self, shape, color, x, y):
self.shape = shape
self.color = color
self.x = x
self.y = y
self.rotation = 0
# 旋转方块
def rotate(self):
self.rotation = (self.rotation + 1) % 4
# 向左移动方块
def move_left(self):
self.x -= BLOCK_SIZE
# 向右移动方块
def move_right(self):
self.x += BLOCK_SIZE
# 向下移动方块
def move_down(self):
self.y += BLOCK_SIZE
# 绘制方块
def draw(self, surface):
for block in self.shape[self.rotation]:
x, y = block
pygame.draw.rect(surface, self.color, (self.x + x * BLOCK_SIZE, self.y + y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 定义游戏主循环
def main():
global score
# 创建一个新方块
block = Block(random.choice(SHAPES), random.choice(COLORS), 120, 0)
# 定义一个布尔值,表示游戏是否结束
game_over = False
# 定义一个二维数组,表示游戏区域
grid = [[None for _ in range(10)] for _ in range(20)]
# 定义一个计时器,用于控制方块的下落速度
clock = pygame.time.Clock()
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
block.move_left()
elif event.key == pygame.K_RIGHT:
block.move_right()
elif event.key == pygame.K_DOWN:
block.move_down()
elif event.key == pygame.K_SPACE:
block.rotate()
# 计算方块下落的速度
fall_speed = 10 + int(score / 100)
# 计时器等待一段时间
clock.tick(fall_speed)
# 将当前方块绘制到游戏窗口中
window.fill((0, 0, 0))
block.draw(window)
# 判断方块是否落到底部或者触碰到其他方块
for block_x, block_y in block.shape[block.rotation]:
x = block.x + block_x * BLOCK_SIZE
y = block.y + block_y * BLOCK_SIZE
if y >= WINDOW_HEIGHT - BLOCK_SIZE or grid[y // BLOCK_SIZE][x // BLOCK_SIZE]:
# 如果方块触碰到其他方块,则将其加入到游戏区域中
for block_x, block_y in block.shape[block.rotation]:
x = block.x + block_x * BLOCK_SIZE
y = block.y + block_y * BLOCK_SIZE
grid[y // BLOCK_SIZE][x // BLOCK_SIZE] = block.color
# 计算得分,并创建一个新方块
for row in range(20):
if all(grid[row]):
score += 10
grid.pop(row)
grid.insert(0, [None for _ in range(10)])
block = Block(random.choice(SHAPES), random.choice(COLORS), 120, 0)
# 判断游戏是否结束
if any(grid[0]):
game_over = True
break
# 绘制游戏区域中的方块
for row in range(20):
for col in range(10):
color = grid[row][col]
if color:
pygame.draw.rect(window, color, (col * BLOCK_SIZE, row * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制得分文本
text = font.render('Score: {}'.format(score), True, (255, 255, 255))
window.blit(text, (10, 10))
# 更新游戏窗口
pygame.display.update()
# 退出 Pygame 库
pygame.quit()
if __name__ == '__main__':
main()
```
这个示例代码中实现了方块的移动、旋转、下落,以及游戏得分和游戏结束的判断等功能。你可以运行代码来查看游戏效果,并根据自己的需要进行修改和完善。
阅读全文
相关推荐
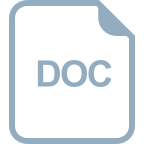
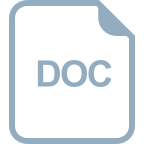
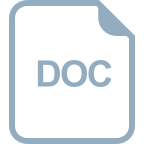












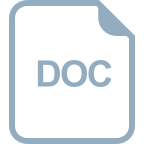
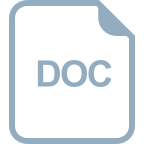