用python写俄罗斯方块??
时间: 2023-08-22 16:06:31 浏览: 235
是的,你可以用 Python 编写俄罗斯方块游戏。以下是一些实现该游戏的基本步骤:
1. 导入 Pygame 库以处理游戏的图形界面。
2. 创建游戏窗口并设置其大小。
3. 创建俄罗斯方块的不同形状并定义它们的旋转动作。
4. 定义方块的运动规则,包括向左移动、向右移动、向下移动和旋转。
5. 定义游戏的主循环,其中包括方块的运动和碰撞检测。
6. 定义游戏的得分系统,包括当一行被填满时,消除该行并增加得分。
7. 定义游戏结束的条件,例如当方块堆叠到屏幕的顶部时游戏结束。
这只是实现俄罗斯方块游戏的基本步骤,你可以根据自己的需要添加其他功能或改进游戏的表现。
相关问题
使用Python写俄罗斯方块
好的,我可以为您提供一些使用Python编写俄罗斯方块的基本思路:
首先,我们需要用Python来实现游戏的图形界面,这可以通过Pygame、Tkinter等库来实现。
其次,我们需要定义俄罗斯方块的各种形状,可以使用数组或者其他数据结构来表示。
然后,我们需要定义游戏的逻辑,包括方块如何下落、旋转、移动等等。
最后,我们可以添加一些额外的功能,比如计分、游戏难度等等。
希望这些信息可以帮助您成功地编写出俄罗斯方块程序!
用python写俄罗斯方块
作为一名 AI,我并没有身体,因此不会打哈欠。但是,我可以为您提供一些关于打哈欠的信息和建议。
打哈欠是一种自然反应,通常是因为身体需要更多氧气或者需要排出二氧化碳。打哈欠也可能是因为疲劳、压力或者缺乏睡眠。
如果您经常打哈欠,可以考虑以下几点建议:
1. 保持良好的睡眠习惯。每晚睡眠时间应该在7-9小时之间,保持规律的睡眠时间可以帮助您更好地休息和恢复。
2. 注意饮食和水分摄入。饮食应该均衡,包括蛋白质、碳水化合物、脂肪和维生素等营养物质。同时,保持足够的水分摄入可以帮助身体保持水平衡,减少疲劳和打哈欠的情况。
3. 适当运动。适当的运动可以帮助身体保持健康,增强身体的免疫力和耐力,减少疲劳和打哈欠的情况。
4. 放松身心。压力和紧张是导致打哈欠的常见原因之一。适当的放松和休息可以帮助您减少压力和紧张,缓解疲劳和打哈欠的情况。
总之,打哈欠是一种自然反应,但如果经常出现,可能是身体需要更多休息和恢复的信号。通过保持良好的睡眠习惯、注意饮食和水分摄入、适当运动和放松身心,可以帮助您减少打哈欠的情况,保持身体健康和精力充沛。
阅读全文
相关推荐
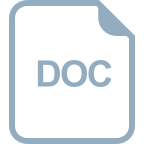
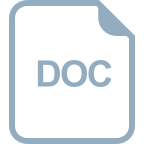
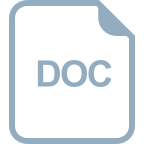










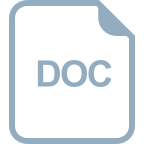
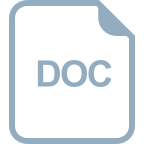
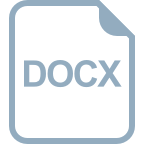