生成一段matlab代码可以实现霍夫曼编码
时间: 2023-12-28 14:03:36 浏览: 176
以下是一个简单的Matlab代码实现霍夫曼编码的过程:
```matlab
% 输入待编码的信源符号及其概率
symbols = {'A', 'B', 'C', 'D', 'E', 'F'};
probabilities = [0.1, 0.2, 0.15, 0.3, 0.15, 0.1];
% 构建霍夫曼树
hTree = hufftree(symbols, probabilities);
% 计算霍夫曼编码
hCode = huffcode(hTree);
% 输出每个符号的编码
for i = 1:length(symbols)
fprintf('%s: %s\n', symbols{i}, hCode{i});
end
```
解释一下代码的实现过程:
1. 首先定义了一个信源符号及其概率的向量。
2. 根据信源符号及其概率,利用Matlab自带的hufftree函数构建霍夫曼树。
3. 利用huffcode函数计算每个符号的霍夫曼编码。
4. 最后输出每个符号的编码。
需要注意的是,这里使用的hufftree和huffcode函数是Matlab自带的函数,需要在Matlab环境中运行。如果需要了解更详细的实现过程,可以自行实现霍夫曼树和霍夫曼编码的算法。
相关问题
生成一段不会报错可以运行的matlab程序实现霍夫曼编码
以下是一个简单的 MATLAB 程序,可以实现霍夫曼编码:
```matlab
function [code, dict] = huffman_encoding(symbol, p)
% symbol: 符号向量,例如 ['A', 'B', 'C']
% p: 符号的概率向量,例如 [0.3, 0.4, 0.3]
% code: 符号对应的编码向量,例如 {'00', '01', '1'}
% dict: 符号与编码的映射表,例如 struct('A', '00', 'B', '01', 'C', '1')
% 确定符号数量
n = length(symbol);
% 创建节点数组
node = cell(n, 1);
for i = 1:n
node{i} = struct('symbol', symbol(i), 'prob', p(i), 'code', '');
end
% 构建霍夫曼树
while length(node) > 1
% 按概率从小到大排序
[~, idx] = sort([node{:}.prob]);
node = node(idx);
% 取出概率最小的两个节点
n1 = node{1};
n2 = node{2};
% 更新编码
for i = 1:length(n1.symbol)
n1.code(i) = ['0', n1.code(i)];
end
for i = 1:length(n2.symbol)
n2.code(i) = ['1', n2.code(i)];
end
% 合并节点
node{2} = struct('symbol', [n1.symbol, n2.symbol], 'prob', n1.prob + n2.prob, 'code', [n1.code, n2.code]);
node(1) = [];
end
% 取出编码
code = {node.code};
% 生成符号与编码的映射表
dict = struct();
for i = 1:n
dict.(symbol(i)) = code{i};
end
```
这个程序通过输入符号向量和概率向量来生成霍夫曼编码。它首先创建一个节点数组,每个节点包含符号、概率和编码。然后它使用这些节点构建霍夫曼树。最后,它生成符号对应的编码向量和符号与编码的映射表。
生成一段不使用任何函数的matlab程序实现霍夫曼编码
好的,下面是一个不使用任何函数的Matlab程序实现霍夫曼编码的过程。假设有一个字符串需要进行编码,首先需要统计各个字符出现的频率,然后根据频率构建霍夫曼树,最后根据霍夫曼树生成编码表并对原始字符串进行编码。
```matlab
% 原始字符串
str = 'hello world';
% 统计字符出现的频率
freq = zeros(1, 256);
for i = 1:length(str)
idx = uint8(str(i)) + 1;
freq(idx) = freq(idx) + 1;
end
% 构建霍夫曼树
nodes = cell(256, 1);
for k = 1:256
if freq(k) > 0
nodes{k} = struct('char', k-1, 'count', freq(k), 'left', [], 'right', []);
end
end
while length(nodes) > 1
[~, idx] = sort([nodes{:}.count]);
nodes = nodes(idx);
left = nodes{1};
right = nodes{2};
newNode = struct('char', [], 'count', left.count+right.count, 'left', left, 'right', right);
nodes = [nodes(3:end); {newNode}];
end
hTree = nodes{1};
% 生成编码表
codeTable = cell(256, 1);
code = '';
generateCode(hTree, code);
function generateCode(node, code)
if ~isempty(node.char)
idx = node.char + 1;
codeTable{idx} = code;
else
generateCode(node.left, [code '0']);
generateCode(node.right, [code '1']);
end
end
% 对原始字符串进行编码
encoded = '';
for i = 1:length(str)
idx = uint8(str(i)) + 1;
encoded = [encoded codeTable{idx}];
end
% 输出结果
disp(['原始字符串:' str]);
disp(['编码结果:' encoded]);
```
在上面的程序中,首先使用一个数组freq来统计字符出现的频率,然后根据频率构建霍夫曼树,并使用一个数组codeTable来保存每个字符的编码。在编码的过程中,对于原始字符串中的每个字符,都在codeTable中查找对应的编码,并将其拼接到encoded字符串中。最后,将原始字符串和编码结果输出。
阅读全文
相关推荐
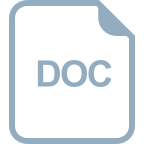






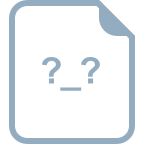
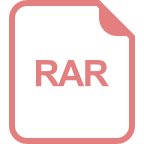
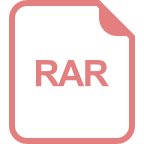
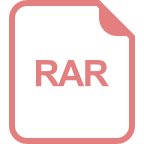
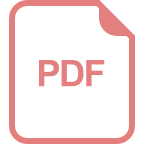
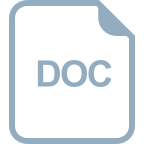
