怎么把xlsx文件转化为ftl文件? java
时间: 2024-09-29 07:15:51 浏览: 74
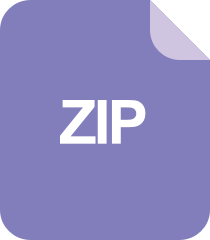
Java POI 生成Excel(xlsx)文件

将Excel(.xlsx)文件转换为FreeMarker(FTL)模板通常需要两步操作:首先读取Excel数据,然后将这些数据写入FTL文件以便动态渲染。以下是使用Java的一个简化示例,使用Apache POI处理Excel和FreeMarker库来完成这个过程:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.velocity.app.VelocityEngine;
import freemarker.template.Configuration;
import java.io.*;
// 1. 读取Excel文件
public void excelToFtl(String inputXlsxPath, String outputFtlPath) throws IOException {
FileInputStream inputStream = new FileInputStream(inputXlsxPath);
Workbook workbook = new XSSFWorkbook(inputStream);
// 从工作簿获取第一个sheet
Sheet sheet = workbook.getSheetAt(0);
// 2. 创建Velocity引擎和FreeMarker配置
VelocityEngine velocityEngine = new VelocityEngine();
Configuration config = new Configuration(Configuration.VERSION_2_3_24);
config.setDirectoryForTemplateLoading(new File("/path/to/templates")); // 指定FTL模板目录
// 3. 遍历Excel数据并生成FTL字符串
try (BufferedWriter writer = new BufferedWriter(new FileWriter(outputFtlPath))) {
for (Row row : sheet) {
Map<String, Object> data = new HashMap<>();
for (int i = 0; i < row.getLastCellNum(); i++) {
Cell cell = row.getCell(i);
switch (cell.getCellType()) { // 根据单元格类型处理数据
case STRING:
data.put(cell.getStringCellValue(), cell.getStringCellValue());
break;
// 其他数据类型如Integer, Date等,类似处理
}
}
String ftlContent = config.getTemplate("template.ftl").render(data); // 使用模板并注入数据
writer.write(ftlContent);
}
}
// 关闭资源
workbook.close();
inputStream.close();
}
阅读全文
相关推荐
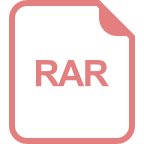
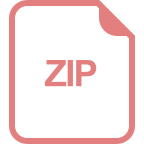
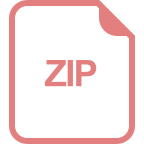
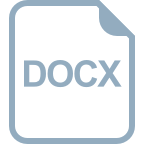
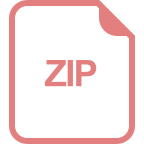
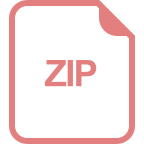
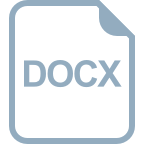
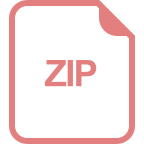
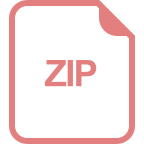
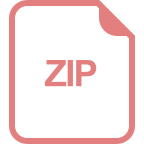
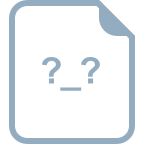
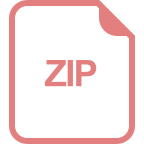
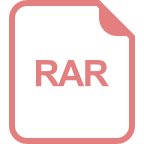
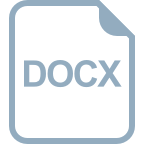
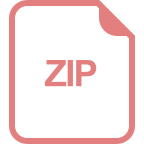
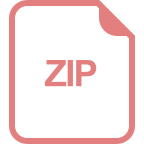
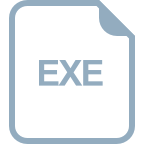