写一段实现PID控制的C++代码
时间: 2024-09-27 20:15:45 浏览: 6
在C++中,实现PID控制器的基本结构通常包括一个类,该类包含输入、输出以及更新控制输出的函数。以下是一个简单的示例,假设我们有一个PID类,用于控制一个线性系统的输出:
```cpp
#include <iostream>
#include <cmath>
class PIDController {
private:
float Kp; // 比例系数
float Ki; // 积分系数
float Kd; // 微分系数
float error; // 当前误差
float integral; // 积分累加
float derivative; // 微分计算
float sample_time; // 更新间隔时间
public:
PIDController(float kp, float ki, float kd, float sample_time = 0.01f) {
Kp = kp;
Ki = ki;
Kd = kd;
this->sample_time = sample_time;
reset();
}
void update(float input, float desired_output) {
error = desired_output - input;
// 更新积分
integral += error * sample_time;
// 计算微分
if (sample_time > 0)
derivative = (error - last_error) / sample_time;
else
derivative = 0;
// 控制输出
output = Kp * error + Ki * integral + Kd * derivative;
last_error = error;
}
float getOutput() { return output; } // 返回当前输出
void reset() {
error = 0;
integral = 0;
derivative = 0;
last_error = 0;
}
};
// 使用示例
int main() {
PIDController pid(1.0, 0.1, 0.05);
// 设定目标值和输入值
float desired_output = 50;
float input = 45;
while (true) {
pid.update(input, desired_output);
std::cout << "Current Output: " << pid.getOutput() << std::endl;
// 这里可以根据pid输出调整实际操作
// 如:input = pid.getOutput();
}
return 0;
}
```
请注意,这只是一个基本示例,实际应用中可能需要处理更复杂的边缘情况,并针对特定硬件平台进行适当的调整。
相关推荐
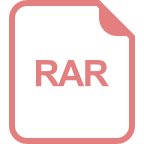
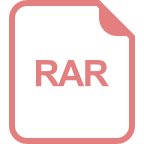
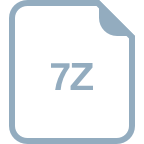














