定义自己的string类mystring
时间: 2023-06-16 13:05:45 浏览: 86
好的,下面是一个简单的实现:
```c++
#include <cstring>
#include <iostream>
class mystring {
private:
char* str;
size_t len;
public:
mystring() : str(nullptr), len(0) {}
mystring(const char* s) : str(nullptr), len(0) {
if (s != nullptr) {
len = std::strlen(s);
str = new char[len + 1];
std::strcpy(str, s);
}
}
mystring(const mystring& other) : str(nullptr), len(other.len) {
if (other.str != nullptr) {
str = new char[len + 1];
std::strcpy(str, other.str);
}
}
~mystring() {
if (str != nullptr) {
delete[] str;
}
}
mystring& operator=(const mystring& other) {
if (this != &other) {
delete[] str;
len = other.len;
if (other.str != nullptr) {
str = new char[len + 1];
std::strcpy(str, other.str);
} else {
str = nullptr;
}
}
return *this;
}
friend std::ostream& operator<<(std::ostream& os, const mystring& s) {
return os << s.str;
}
size_t length() const {
return len;
}
const char* c_str() const {
return str;
}
};
```
这是一个简单的 `mystring` 类,它具有以下功能:
- 默认构造函数和析构函数。
- 从 C 字符串构造函数。
- 拷贝构造函数。
- 赋值运算符。
- `length()` 成员函数,返回字符串长度。
- `c_str()` 成员函数,返回 C 字符串。
示例用法:
```c++
int main() {
mystring s1{"Hello world!"};
mystring s2{s1};
mystring s3;
s3 = s2;
std::cout << s1 << std::endl;
std::cout << s2 << std::endl;
std::cout << s3 << std::endl;
std::cout << "Length of s1: " << s1.length() << std::endl;
std::cout << "C string of s2: " << s2.c_str() << std::endl;
return 0;
}
```
输出:
```
Hello world!
Hello world!
Hello world!
Length of s1: 12
C string of s2: Hello world!
```
阅读全文
相关推荐




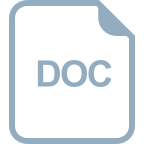
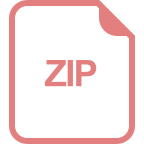








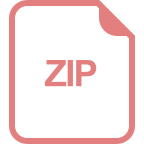