插入排序、堆排序、快速排序、冒泡排序,请用c++语言实现上述排序,写在同一个程序里
时间: 2023-08-19 10:03:46 浏览: 81
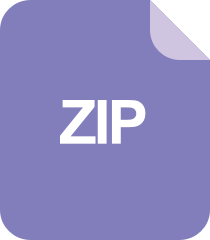
排序算法(插入、选择、归并、冒泡、堆排序)实现代码C++

下面是对应的 C++ 代码实现,包括插入排序、堆排序、快速排序和冒泡排序:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 插入排序
void insertionSort(vector<int>& arr) {
int n = arr.size();
for (int i = 1; i < n; ++i) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
// 堆排序
void heapSort(vector<int>& arr) {
make_heap(arr.begin(), arr.end());
sort_heap(arr.begin(), arr.end());
}
// 快速排序
int partition(vector<int>& arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; ++j) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return i + 1;
}
void quickSort(vector<int>& arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// 冒泡排序
void bubbleSort(vector<int>& arr) {
int n = arr.size();
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
}
}
}
}
int main() {
vector<int> arr = { 3, 7, 1, 4, 9, 2, 6, 5, 8 };
cout << "Original Array: ";
for (auto num : arr) {
cout << num << " ";
}
cout << endl;
// 插入排序
vector<int> arr1 = arr;
insertionSort(arr1);
cout << "After Insertion Sort: ";
for (auto num : arr1) {
cout << num << " ";
}
cout << endl;
// 堆排序
vector<int> arr2 = arr;
heapSort(arr2);
cout << "After Heap Sort: ";
for (auto num : arr2) {
cout << num << " ";
}
cout << endl;
// 快速排序
vector<int> arr3 = arr;
quickSort(arr3, 0, arr3.size() - 1);
cout << "After Quick Sort: ";
for (auto num : arr3) {
cout << num << " ";
}
cout << endl;
// 冒泡排序
vector<int> arr4 = arr;
bubbleSort(arr4);
cout << "After Bubble Sort: ";
for (auto num : arr4) {
cout << num << " ";
}
cout << endl;
return 0;
}
```
这段程序首先定义了四个排序函数:插入排序、堆排序、快速排序和冒泡排序。然后,程序创建了一个整数数组 `arr`,并使用它对这四个排序函数进行测试,输出排序后的结果。
阅读全文
相关推荐
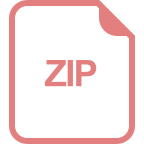
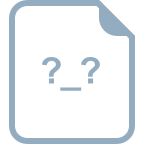
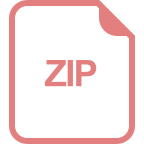
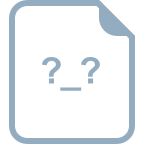
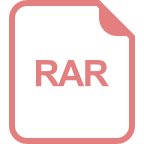
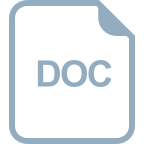







