使用STM32F103芯片编写一个访问DS18B20程序
时间: 2024-05-07 13:15:58 浏览: 111
以下为使用STM32F103芯片编写访问DS18B20程序的示例代码:
```c
#include "stm32f10x.h"
#define DS18B20_PIN GPIO_Pin_15
#define DS18B20_PORT GPIOB
void delay_us(uint32_t us)
{
for(uint32_t i=0; i<us*8; i++);
}
void ds18b20_init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStruct.GPIO_Pin = DS18B20_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStruct);
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
}
void ds18b20_write_byte(uint8_t byte)
{
for(uint8_t i=0; i<8; i++) {
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
delay_us(2);
if(byte & (1 << i)) {
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
}
delay_us(60);
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
delay_us(2);
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t byte = 0;
for(uint8_t i=0; i<8; i++) {
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
delay_us(2);
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
delay_us(2);
if(GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN)) {
byte |= (1 << i);
}
delay_us(60);
}
return byte;
}
void ds18b20_start_conversion(void)
{
ds18b20_init();
ds18b20_write_byte(0xCC); // Skip ROM
ds18b20_write_byte(0x44); // Start conversion
}
float ds18b20_read_temperature(void)
{
ds18b20_init();
ds18b20_write_byte(0xCC); // Skip ROM
ds18b20_write_byte(0xBE); // Read scratchpad
uint8_t temp_l = ds18b20_read_byte();
uint8_t temp_h = ds18b20_read_byte();
float temp = ((temp_h << 8) | temp_l) * 0.0625;
return temp;
}
int main(void)
{
float temperature;
ds18b20_start_conversion();
while(1) {
temperature = ds18b20_read_temperature();
// Do something with temperature
}
}
```
上述代码实现了DS18B20的初始化、写入字节、读取字节、启动转换和读取温度等功能。其中,ds18b20_start_conversion()函数用于启动转换,ds18b20_read_temperature()函数用于读取温度。在主函数中,使用while(1)循环不断读取温度并进行处理。注意,由于DS18B20的读取需要一定的时间,因此需要适当延迟一段时间后再进行读取。
阅读全文
相关推荐
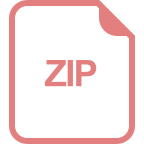
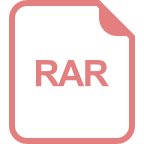
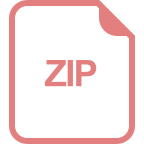
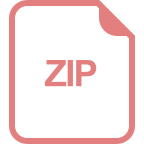

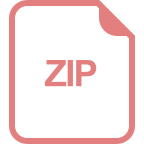
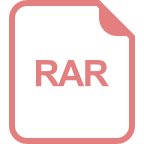
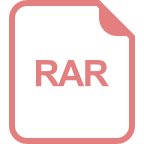
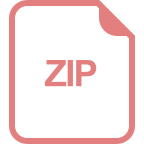
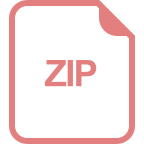
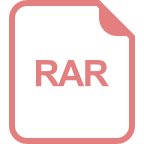
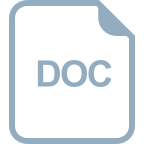
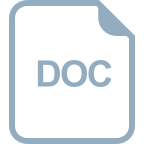
