python宽度优先搜索
时间: 2023-11-09 11:03:30 浏览: 59
宽度优先搜索(BFS)是一种图形搜索算法,用于遍历或搜索树或图的数据结构。它从根节点开始,逐层遍历整个图,直到找到目标节点或遍历完整个图。在实现中,通常使用队列来存储每个节点的状态。
Python中实现BFS的一般步骤如下:
1. 创建一个队列,并将起始节点放入队列中。
2. 从队列中取出第一个节点,并检查它是否为目标节点。如果是,则返回结果并结束搜索。
3. 如果不是目标节点,则将其所有未访问过的邻居节点加入队列中,并将该节点标记为已访问。
4. 重复步骤2和3,直到队列为空或找到目标节点。
以下是一个简单的Python代码示例,用于在二叉树中查找特定值:
```python
class Node:
def __init__(self, value):
self.left = None
self.right = None
self.value = value
def bfs(node, target):
queue = [node]
visited = set()
while queue:
current = queue.pop(0)
if current.value == target:
return True
visited.add(current)
if current.left and current.left not in visited:
queue.append(current.left)
if current.right and current.right not in visited:
queue.append(current.right)
return False
# 示例用法
root = Node(1)
root.left = Node(2)
root.right = Node(3)
root.left.left = Node(4)
root.left.right = Node(5)
print(bfs(root, 5)) # 输出 True
print(bfs(root, 6)) # 输出 False
```
相关推荐
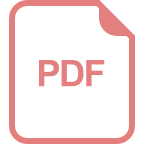
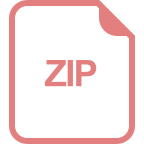














