c语言写非二叉树的插入结点
时间: 2023-07-13 18:31:59 浏览: 54
在C语言中,可以通过链表的方式来实现非二叉树的插入结点操作。以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义树的结点类型
typedef struct TreeNode {
int data; // 结点的数据
struct TreeNode *firstChild; // 第一个孩子结点
struct TreeNode *nextSibling; // 兄弟结点
} TreeNode;
// 插入结点
void insertNode(TreeNode *parent, TreeNode *node) {
if (parent == NULL) {
return;
}
if (parent->firstChild == NULL) {
parent->firstChild = node;
} else {
TreeNode *child = parent->firstChild;
while (child->nextSibling != NULL) {
child = child->nextSibling;
}
child->nextSibling = node;
}
}
int main() {
// 创建根结点
TreeNode *root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = 1;
root->firstChild = NULL;
root->nextSibling = NULL;
// 创建结点2、3、4
TreeNode *node2 = (TreeNode*)malloc(sizeof(TreeNode));
node2->data = 2;
node2->firstChild = NULL;
node2->nextSibling = NULL;
TreeNode *node3 = (TreeNode*)malloc(sizeof(TreeNode));
node3->data = 3;
node3->firstChild = NULL;
node3->nextSibling = NULL;
TreeNode *node4 = (TreeNode*)malloc(sizeof(TreeNode));
node4->data = 4;
node4->firstChild = NULL;
node4->nextSibling = NULL;
// 插入结点2、3、4
insertNode(root, node2);
insertNode(root, node3);
insertNode(node2, node4);
// 输出树的结点
printf("Tree: root(%d)->", root->data);
TreeNode *child = root->firstChild;
while (child != NULL) {
printf("(%d)->", child->data);
child = child->nextSibling;
}
printf("NULL\n");
return 0;
}
```
在上面的例子中,插入结点的操作通过遍历找到最后一个兄弟结点,并将新结点插入到其后面。注意,如果某个结点没有孩子结点,则其 `firstChild` 指针应该被设置为 `NULL`。
相关推荐
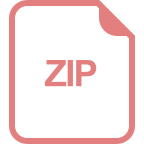
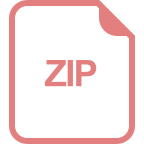
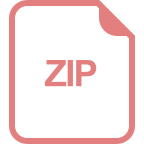
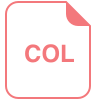
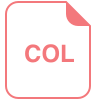












