event == cv.EVENT_LBUTTONDOWN
时间: 2024-09-17 21:03:03 浏览: 39
在OpenCV库中,`cv.EVENT_LBUTTONDOWN`是一个预定义的事件常量,它代表鼠标左键按下事件。当用户点击图像窗口并按住左键时,OpenCV会捕获这个事件。开发者可以注册一个回调函数,该函数会在发生左键单击时被调用,以便根据用户的操作更新图像处理或其他相关的程序逻辑。
在Python的OpenCV脚本中,你可以使用`cv2.setMouseCallback()`函数来设置这样的事件处理器,示例代码如下:
```python
import cv2
def left_button_down(event, x, y, flags, param):
if event == cv2.EVENT_LBUTTONDOWN:
# 在这里添加处理左键点击的代码
print(f"Left button clicked at position ({x}, {y})")
# 设置回调函数
cv2.namedWindow("image")
cv2.setMouseCallback("image", left_button_down)
while True:
# ... (显示图像和其他循环逻辑)
```
相关推荐
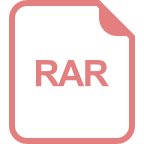
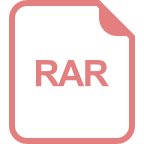
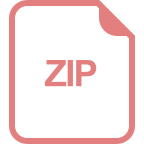
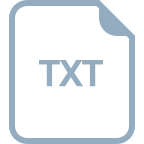
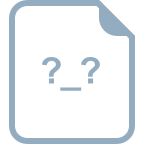
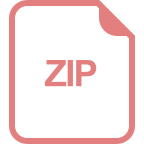
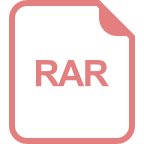
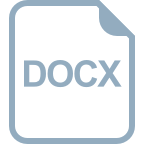
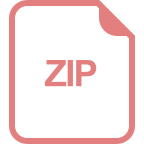
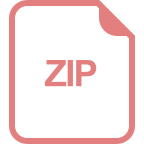
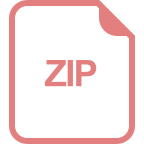