linux rs485编程
时间: 2023-08-01 18:15:30 浏览: 132
RS485是一种串行通信协议,可以用于在较长距离的设备之间进行通信。在Linux系统中,可以使用串口编程库来实现RS485通信。
以下是一个简单的Linux C程序示例,用于发送和接收RS485数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define SERIAL_PORT "/dev/ttyUSB0"
int main(int argc, char *argv[]) {
int fd;
struct termios options;
char buffer[255];
int n;
// 打开串口
fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
fprintf(stderr, "无法打开串口\n");
exit(EXIT_FAILURE);
}
// 配置串口
tcgetattr(fd, &options);
cfmakeraw(&options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
tcsetattr(fd, TCSANOW, &options);
// 发送数据
strcpy(buffer, "Hello, RS485!");
n = write(fd, buffer, strlen(buffer));
if (n < 0) {
fprintf(stderr, "写入数据失败\n");
exit(EXIT_FAILURE);
}
// 读取数据
memset(buffer, 0, sizeof(buffer));
n = read(fd, buffer, sizeof(buffer));
if (n < 0) {
fprintf(stderr, "读取数据失败\n");
exit(EXIT_FAILURE);
}
printf("接收到的数据:%s\n", buffer);
// 关闭串口
close(fd);
return 0;
}
```
在上面的示例中,我们首先使用`open`函数打开串口设备`/dev/ttyUSB0`,然后使用`tcgetattr`和`tcsetattr`函数配置串口参数,包括波特率、数据位、校验位、停止位等。然后我们将要发送的数据写入串口,使用`read`函数读取串口返回的数据。最后使用`close`函数关闭串口。
需要注意的是,RS485是半双工通信协议,发送和接收需要分别进行,不能同时进行。在发送数据时,需要等待数据发送完成后再进行接收,否则可能会出现数据冲突和错误。
阅读全文
相关推荐
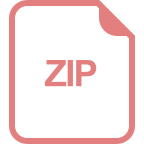
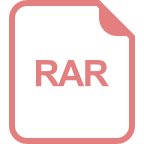















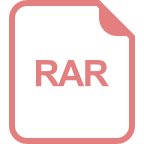