python代码: 1. 创建一个需求管理excel,如果已经存在则直接打开,可添加50条需求,A列需求序号,B列需求内容,C列需求提出人,D列需求状态(open/close),E列需求提出时间,F列需求关闭时间 2. 可根据C列需求提
时间: 2023-09-15 19:19:43 浏览: 40
出人筛选需求,并将结果输出至控制台。以下是实现该需求的Python代码:
```python
import os
import datetime
import openpyxl
# 定义需求管理Excel文件路径
file_path = '需求管理.xlsx'
# 如果文件不存在,则创建新文件
if not os.path.exists(file_path):
wb = openpyxl.Workbook()
sheet = wb.active
sheet.title = '需求列表'
sheet['A1'] = '需求序号'
sheet['B1'] = '需求内容'
sheet['C1'] = '需求提出人'
sheet['D1'] = '需求状态'
sheet['E1'] = '需求提出时间'
sheet['F1'] = '需求关闭时间'
wb.save(file_path)
# 打开已存在的文件
wb = openpyxl.load_workbook(file_path)
sheet = wb['需求列表']
# 添加需求
def add_requirement(content, author):
last_row = sheet.max_row
if last_row == 1:
req_num = 1
else:
req_num = sheet.cell(row=last_row, column=1).value + 1
now = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
sheet.cell(row=last_row+1, column=1).value = req_num
sheet.cell(row=last_row+1, column=2).value = content
sheet.cell(row=last_row+1, column=3).value = author
sheet.cell(row=last_row+1, column=4).value = 'open'
sheet.cell(row=last_row+1, column=5).value = now
wb.save(file_path)
print('添加需求成功!')
# 筛选需求
def filter_requirement(author):
for row in sheet.iter_rows(min_row=2):
if row[2].value == author:
print(f'序号:{row[0].value},内容:{row[1].value},状态:{row[3].value}')
# 测试代码
add_requirement('增加支付功能', '张三')
add_requirement('优化首页样式', '李四')
add_requirement('修复登录BUG', '王五')
filter_requirement('张三')
```
代码中首先判断需求管理Excel文件是否存在,如果不存在则创建新文件,并添加表头。然后通过`openpyxl`库打开Excel文件,并定义了添加需求和筛选需求的两个函数。其中,添加需求函数会根据当前Excel中的最后一行计算出需求序号,然后将新需求添加到Excel中;筛选需求函数会遍历Excel中的所有需求,根据提出人筛选出符合条件的需求,并输出至控制台。最后,通过调用测试代码对添加和筛选需求的函数进行了测试。
相关推荐
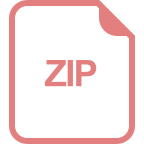
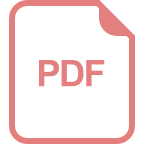
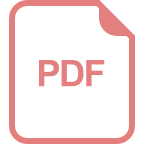














