编写函数计算两个正整数的最小公倍数(Least Common Multiple,LCM),函数原型如下: /* 函数功能:计算a和b的最小公倍数,当a或者b为非正整数时返回-1 */ int Lcm(int a, int b); 然后在主函数中调用该函数计算并输出从键盘任意输入的两整数的最小公倍数。 **提示信息:"Input a,b:" **输入格式要求:"%d,%d" **输出格式要求:"Least Common Multiple of %d and %d is %d\n" "Input error!\n"(输入0或负数时输出) 程序运行示例1如下: Input a,b:16,24 Least Common Multiple of 16 and 24 is 48 程序运行示例2如下: Input a,b:0,24 Input error! 注:不允许使用goto语句
时间: 2024-01-19 11:02:54 浏览: 90
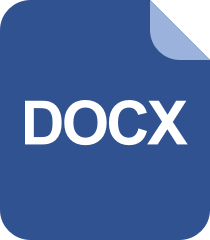
计算两个整数的最小公倍数.docx
下面是代码实现:
```
#include <stdio.h>
int Gcd(int a, int b) {
int temp;
while (b > 0) {
temp = b;
b = a % b;
a = temp;
}
return a;
}
int Lcm(int a, int b) {
if (a <= 0 || b <= 0) {
return -1;
}
return a * b / Gcd(a, b);
}
int main() {
int a, b;
printf("Input a,b:");
scanf("%d,%d", &a, &b);
int lcm = Lcm(a, b);
if (lcm == -1) {
printf("Input error!\n");
} else {
printf("Least Common Multiple of %d and %d is %d\n", a, b, lcm);
}
return 0;
}
```
阅读全文
相关推荐
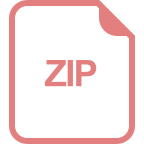
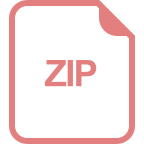















