Python tkinter Listbox
时间: 2023-11-19 12:56:10 浏览: 38
The Listbox widget in tkinter is used to display a list of items. It allows the user to select one or more items from the list. The following code creates a Listbox widget and adds items to it:
```
import tkinter as tk
root = tk.Tk()
# Create a Listbox widget
listbox = tk.Listbox(root)
# Add items to the Listbox
listbox.insert(0, "Item 1")
listbox.insert(1, "Item 2")
listbox.insert(2, "Item 3")
# Pack the Listbox widget
listbox.pack()
root.mainloop()
```
In the above code, we first import the tkinter module and create a new instance of the Tk class. We then create a Listbox widget and add items to it using the `insert` method. Finally, we pack the Listbox widget using the `pack` method and start the main event loop using the `mainloop` method of the root window.
We can also bind a function to the Listbox widget to be executed when an item is selected. The following code demonstrates this:
```
import tkinter as tk
root = tk.Tk()
# Define a function to be called when an item is selected
def on_select(event):
selected_item = event.widget.get(event.widget.curselection())
print("Selected item:", selected_item)
# Create a Listbox widget
listbox = tk.Listbox(root)
# Add items to the Listbox
listbox.insert(0, "Item 1")
listbox.insert(1, "Item 2")
listbox.insert(2, "Item 3")
# Bind the on_select function to the Listbox widget
listbox.bind('<<ListboxSelect>>', on_select)
# Pack the Listbox widget
listbox.pack()
root.mainloop()
```
In the above code, we define a function `on_select` that is called when an item is selected. This function retrieves the selected item using the `curselection` method of the Listbox widget and prints it to the console. We then bind this function to the Listbox widget using the `bind` method and the `<<ListboxSelect>>` event. When an item is selected, the `on_select` function is called and the selected item is printed to the console.
相关推荐
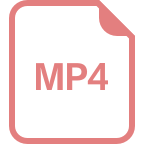














