python实现遗传算法tsp旅行商问题
时间: 2023-12-04 14:39:19 浏览: 115
以下是Python实现遗传算法解决TSP旅行商问题的示例代码:
```python
import random
# 城市坐标
city_pos = [(60, 200), (180, 200), (80, 180), (140, 180), (20, 160), (100, 160), (200, 160), (140, 140), (40, 120), (100, 120), (180, 100), (60, 80), (120, 80), (180, 60), (20, 40), (100, 40), (200, 40), (20, 20), (60, 20), (160, 20)]
# 种群大小
POP_SIZE = 500
# 城市数量
ITY_COUNT = len(city_pos)
# 交叉概率
CROSS_RATE = 0.1
# 变异概率
MUTATION_RATE = 0.02
# 代数
N_GENERATIONS = 500
# 计算两个城市之间的距离
def distance(city1, city2):
return ((city1[0] - city2[0]) ** 2 + (city1[1] - city2[1]) ** 2) ** 0.5
# 计算一条路径的总距离
def get_fitness(path):
distance_sum = 0
for i in range(CITY_COUNT - 1):
distance_sum += distance(city_pos[path[i]], city_pos[path[i+1]])
distance_sum += distance(city_pos[path[-1]], city_pos[path[0]])
return 1 / distance_sum
# 初始化种群
def init_population():
population = []
for i in range(POP_SIZE):
path = list(range(CITY_COUNT))
random.shuffle(path)
population.append(path)
return population
# 选择
def select(population, fitness):
idx = random.randint(0, POP_SIZE - 1)
for i in range(POP_SIZE):
if random.random() < fitness[i] / fitness.sum():
idx = i
break
return population[idx]
# 交叉
def crossover(parent1, parent2):
if random.random() < CROSS_RATE:
child = [-1] * CITY_COUNT
start = random.randint(0, CITY_COUNT - 1)
end = random.randint(start, CITY_COUNT - 1)
child[start:end+1] = parent1[start:end+1]
for i in range(CITY_COUNT):
if parent2[i] not in child:
for j in range(CITY_COUNT):
if child[j] == -1:
child[j] = parent2[i]
break
return child
else:
return parent1
# 变异
def mutate(child):
if random.random() < MUTATION_RATE:
idx1, idx2 = random.sample(range(CITY_COUNT), 2)
child[idx1], child[idx2] = child[idx2], child[idx1]
return child
# 遗传算法主函数
def genetic_algorithm():
population = init_population()
for generation in range(N_GENERATIONS):
fitness = [get_fitness(path) for path in population]
best_path = population[fitness.index(max(fitness))]
print("Generation:", generation, "| Best path length:", 1 / max(fitness))
new_population = [best_path]
for i in range(POP_SIZE - 1):
parent1 = select(population, fitness)
parent2 = select(population, fitness)
child = crossover(parent1, parent2)
child = mutate(child)
new_population.append(child)
population = new_population
return best_path
# 运行遗传算法
best_path = genetic_algorithm()
print("Best path:", best_path)
```
阅读全文
相关推荐
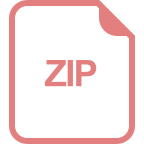
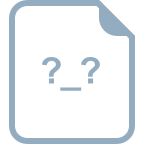
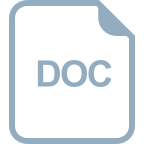
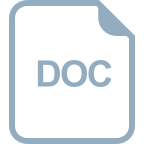

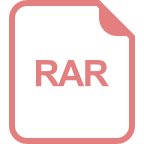
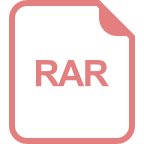
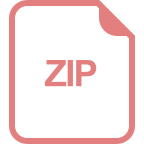
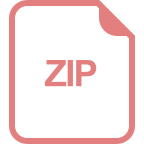
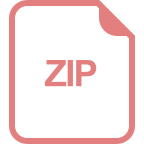
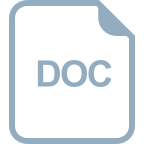
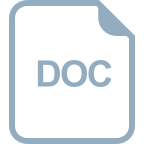
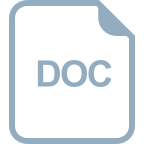
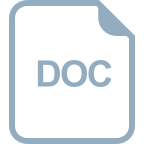

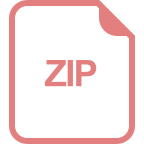