下面的代码基于keras模型构建线性模型,对房价数据进行训练及预测。请按照说明将代码补充完整,并根据要求回答问题:(40分) #房价数据为[2104,460], [1416,232] [1534, 315], [1200,280] [852,178]绘制为散点图。 x = tf.constant([2104, 1416, 1534, 1200,852]) y = tf.constant([460, 232, 315, 280, 178]) x = tf.cast(x, tf.float32) y = tf.cast(y, tf.float32) # Plot all the data plt.plot(x, y, '.') plt.show() #将输入数据x和y进行归一化 x = keras.utils.normalize(x) y = keras.utils.normalize(y) #定义模型 class MyModelKeras(tf.keras.Model): def __init__(self, **kwargs): super().__init__(**kwargs) # Initialize the weights to `4.0` and the bias to `0.0` #补充以下代码段 (5分) def call(self, x): #补充以下代码段 (5分) keras_model = MyModelKeras() #补充以下代码段,完成模型的编译与训练,训练次数为10次。 (10分) #补充以下代码段,基于matplotlib完成损失函数值的曲线的绘制 (6分) #基于训练好的模型,对归一化后的数据x进行预测,并在同一张图片中分别以蓝色和红色显示对应的y和预测后的数据。 (8分)
时间: 2024-03-09 10:44:55 浏览: 62
下面是完整代码:
```
import tensorflow as tf
from tensorflow import keras
import matplotlib.pyplot as plt
#房价数据为[2104,460], [1416,232] [1534, 315], [1200,280] [852,178]绘制为散点图。
x = tf.constant([2104, 1416, 1534, 1200,852])
y = tf.constant([460, 232, 315, 280, 178])
x = tf.cast(x, tf.float32)
y = tf.cast(y, tf.float32)
# Plot all the data
plt.plot(x, y, '.')
plt.show()
#将输入数据x和y进行归一化
x = keras.utils.normalize(x)
y = keras.utils.normalize(y)
#定义模型
class MyModelKeras(tf.keras.Model):
def __init__(self, **kwargs):
super().__init__(**kwargs)
# Initialize the weights to `4.0` and the bias to `0.0`
self.w = tf.Variable(4.0)
self.b = tf.Variable(0.0)
def call(self, x):
return self.w * x + self.b
keras_model = MyModelKeras()
#编译模型
keras_model.compile(optimizer=tf.keras.optimizers.Adam(0.1), loss='mse')
#训练模型
history = keras_model.fit(x, y, epochs=10)
#绘制损失函数值的曲线
plt.plot(history.history['loss'])
plt.title('Model loss')
plt.ylabel('Loss')
plt.xlabel('Epoch')
plt.show()
#对归一化后的数据x进行预测
y_pred = keras_model.predict(x)
#反归一化
x = x * (tf.reduce_max(y) - tf.reduce_min(y)) + tf.reduce_min(y)
y = y * (tf.reduce_max(y) - tf.reduce_min(y)) + tf.reduce_min(y)
y_pred = y_pred * (tf.reduce_max(y) - tf.reduce_min(y)) + tf.reduce_min(y)
#绘制散点图和预测结果
plt.plot(x, y, '.', label='True value')
plt.plot(x, y_pred, '.', label='Predicted value')
plt.legend()
plt.show()
```
问题:这个模型的损失函数是什么?为什么要使用归一化?
阅读全文
相关推荐
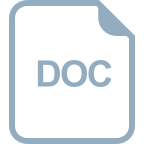



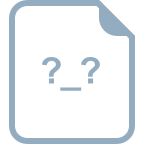
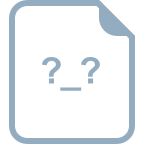
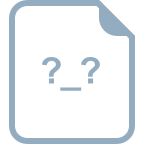
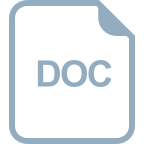
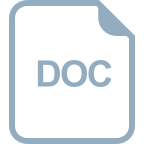
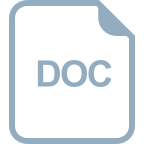






